5.4.5.2 Use CMSIS ITM Functions
Right click on the project name in the Projects window and select “Properties.” Under Packs, in addition to the device pack (DFP), CMSIS is also included (see figure below).
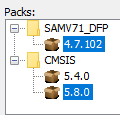
The Common Microcontroller Software Interface Standard (CMSIS) is a vendor-independent abstraction layer for microcontrollers that are based on Arm Cortex processors. CMSIS defines generic tool interfaces and enables consistent device support. The CMSIS software interfaces simplify software reuse, reduce the learning curve for microcontroller developers, and improve time to market for new devices.
The ARM CMSIS package comes with specific header files/APIs for sending ITM data. For example, in <MPLAB_Installation>packs\arm\CMSIS\x.x.x\CMSIS\Core\Include\core_cm7.h,
find the CMSIS function ITM_SendChar()
that can be used to print a
character over ITM/SWO:
__STATIC_INLINE uint32_t ITM_SendChar(uint32_t ch)
These are the lowest level APIs at the byte level (only writing to PORT 0); however you can customize and develop your own functions for printing the messages to any port 0 through 31 (see 5.4 ARM ITM/SWO Trace).
__STATIC_INLINE uint32_t ITM_SendCharPort (uint8_t port, uint32_t ch)
{
if (((ITM->TCR & ITM_TCR_ITMENA_Msk) != 0UL) && /* ITM enabled */
((ITM->TER & 1UL << port ) != 0UL) ) /* ITM Port enabled */
{
while (ITM->PORT[port].u32 == 0UL)
{
__NOP();
}
ITM->PORT[port].u8 = (uint8_t)ch;
}
return (ch);
}
//-SL: same as ITM-fct from CMSIS-header (see above), but with portNum
void ITM_PrintString(const char *s, uint8_t portNo)
{
while (*s!='\0')
{
ITM_SendCharPort(portNo,*s++);
}
}
Alternately, if you do not want to use CMSIS ITM functions, you can write your own by understanding the ITM PORT registers and ITM configuration/status registers which are available as part of the ARM CoreSight Documentation.