3.16 PWM Driver
Overview
PWM is used to generate the PWM pulses, this PWM is used to drive motor and other applications.
Features
- PWM Frequency and the Duty cycle is user configurable, the range of the Frequency value depends on the clock source selected from the Hardware Dependency module (PLIB).
- Internal timer operates in Synchronization (Sync) or Trigger mode.
- Sync sources are user configurable, the timer Reset or clear occurs when the input selected by the Sync source is asserted. The Timer immediately begins to count again from zero unless it is held for some other reason.
- In Trigger operation, the timer is held in Reset until the input selected by Sync source is asserted.
The following waveform represents the PWM output and the effect of Sync source.
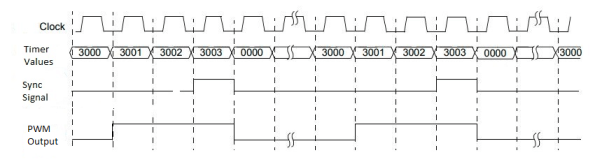
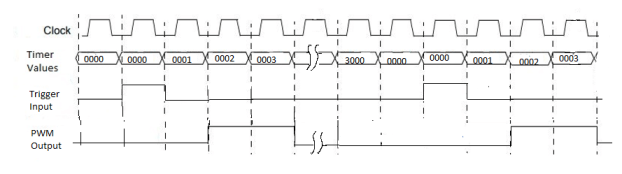
3.16.1 Module Documentation
3.16.1.1 PWM Driver
Pulse Width Modulation Driver using dsPIC MCUs.
3.16.1.1.1 Module description
Pulse Width Modulation Driver using dsPIC MCUs.
Data structures
struct PWM_INTERFACE
Structure containing the function pointers of PWM generator driver.
Definitions
#define PWM1_Initialize SCCP1_PWM_Initialize
This macro defines the Custom Name for SCCP1_PWM_Initialize API.
#define PWM1_Deinitialize SCCP1_PWM_Deinitialize
This macro defines the Custom Name for SCCP1_PWM_Deinitialize API.
#define PWM1_Enable SCCP1_PWM_Enable
This macro defines the Custom Name for SCCP1_PWM_Enable API.
#define PWM1_Disable SCCP1_PWM_Disable
This macro defines the Custom Name for SCCP1_PWM_Disable API.
#define PWM1_PeriodSet SCCP1_PWM_PeriodSet
This macro defines the Custom Name for SCCP1_PWM_PeriodSet API.
#define PWM1_DutyCycleSet SCCP1_PWM_DutyCycleSet
This macro defines the Custom Name for SCCP1_PWM_DutyCycleSet API.
#define PWM1_SoftwareTriggerSet SCCP1_PWM_SoftwareTriggerSet
This macro defines the Custom Name for SCCP1_PWM_SoftwareTriggerSet API.
#define PWM1_Tasks SCCP1_PWM_Tasks
This macro defines the Custom Name for SCCP1_PWM_Tasks API.
#define PWM1_PWM_CallbackRegister SCCP1_PWM_CallbackRegister
This macro defines the Custom Name for SCCP1_PWM_CallbackRegister API.
Enumerations
enum PWM_OUTPUT_MODES { OUTPUT_MODES_NOT_AVAILABLE }
Enumeration containing the output modes of PWM driver.
Functions
void SCCP1_PWM_Initialize (void)
Initializes the SCCP1 Pulse driver. This function must be called before any other SCCP1 function is called.
void SCCP1_PWM_Deinitialize (void)
Deinitializes the SCCP1 to POR values.
void SCCP1_PWM_Enable (void)
Enables the SCCP1 module.
void SCCP1_PWM_Disable (void)
Disables the SCCP1 module.
void SCCP1_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
void SCCP1_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
void SCCP1_PWM_SoftwareTriggerSet (void)
This function sets the manual trigger.
void SCCP1_PWM_CallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for SCCP1 PWM event.
void SCCP1_PWM_Callback (void)
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using SCCP1_PWM_CallbackRegister.
void SCCP1_PWM_Tasks (void)
This function is used to implement the tasks for polled implementations.
Variables
const struct PWM_INTERFACE PWM1
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface. The default name e.g. PWM1 can be changed by the user in the MCCP user interface. This allows defining a structure with application specific name using the 'Custom Name' field. Application specific name allows the API Portability.
3.16.1.1.2 Definition Documentation
PWM1_Deinitialize
#define PWM1_Deinitialize SCCP1_PWM_Deinitialize
This macro defines the Custom Name for SCCP1_PWM_Deinitialize API.
PWM1_Disable
#define PWM1_Disable SCCP1_PWM_Disable
This macro defines the Custom Name for SCCP1_PWM_Disable API.
PWM1_DutyCycleSet
#define PWM1_DutyCycleSet SCCP1_PWM_DutyCycleSet
This macro defines the Custom Name for SCCP1_PWM_DutyCycleSet API.
PWM1_Enable
#define PWM1_Enable SCCP1_PWM_Enable
This macro defines the Custom Name for SCCP1_PWM_Enable API.
PWM1_Initialize
#define PWM1_Initialize SCCP1_PWM_Initialize
This macro defines the Custom Name for SCCP1_PWM_Initialize API.
PWM1_PeriodSet
#define PWM1_PeriodSet SCCP1_PWM_PeriodSet
This macro defines the Custom Name for SCCP1_PWM_PeriodSet API.
PWM1_PWM_CallbackRegister
#define PWM1_PWM_CallbackRegister SCCP1_PWM_CallbackRegister
This macro defines the Custom Name for SCCP1_PWM_CallbackRegister API.
PWM1_SoftwareTriggerSet
#define PWM1_SoftwareTriggerSet SCCP1_PWM_SoftwareTriggerSet
This macro defines the Custom Name for SCCP1_PWM_SoftwareTriggerSet API.
PWM1_Tasks
#define PWM1_Tasks SCCP1_PWM_Tasks
This macro defines the Custom Name for SCCP1_PWM_Tasks API.
3.16.1.1.3 Function Documentation
SCCP1_PWM_Callback()
void SCCP1_PWM_Callback (void )
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using SCCP1_PWM_CallbackRegister.
none |
none |
SCCP1_PWM_CallbackRegister()
void SCCP1_PWM_CallbackRegister (void(*)(void) handler)
This function can be used to override default callback and to define custom callback for SCCP1 PWM event.
in | handler |
- Address of the callback function |
none |
SCCP1_PWM_Deinitialize()
void SCCP1_PWM_Deinitialize (void )
Deinitializes the SCCP1 to POR values.
none |
none |
SCCP1_PWM_Disable()
void SCCP1_PWM_Disable (void )
Disables the SCCP1 module.
none |
none |
SCCP1_PWM_DutyCycleSet()
void SCCP1_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
in | dutyCycleCount |
- number of cycles of ON time |
none |
SCCP1_PWM_Enable()
void SCCP1_PWM_Enable (void )
Enables the SCCP1 module.
none |
none |
SCCP1_PWM_Initialize()
void SCCP1_PWM_Initialize (void )
Initializes the SCCP1 Pulse driver. This function must be called before any other SCCP1 function is called.
none |
none |
SCCP1_PWM_PeriodSet()
void SCCP1_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
in | periodCount |
- number of clock counts for PWM Period |
none |
SCCP1_PWM_SoftwareTriggerSet()
void SCCP1_PWM_SoftwareTriggerSet (void )
This function sets the manual trigger.
none |
none |
SCCP1_PWM_Tasks()
void SCCP1_PWM_Tasks (void )
This function is used to implement the tasks for polled implementations.
none |
none |
3.16.1.1.4 Enumeration Type Documentation
PWM_OUTPUT_MODES
enum PWM_OUTPUT_MODES
Enumeration containing the output modes of PWM driver.
OUTPUT_MODES_NOT_AVAILABLE |
No output modes available |
3.16.1.1.5 Variable Documentation
PWM1
const struct PWM_INTERFACE PWM1
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface. The default name e.g. PWM1 can be changed by the user in the MCCP user interface. This allows defining a structure with application specific name using the 'Custom Name' field. Application specific name allows the API Portability.
3.16.2 Data Structure Documentation
3.16.2.1 PWM_INTERFACE Struct Reference
Structure containing the function pointers of PWM generator driver.
3.16.2.1.1 Detailed Description
Structure containing the function pointers of PWM generator driver.
#include <pwm_interface.h>
Data Fields
void(* Initialize )(void)
Pointer to SCCPx_PWM_Initialize e.g. SCCP1_PWM_Initialize.
void(* Deinitialize )(void)
Pointer to SCCPx_PWM_Deinitialize e.g. SCCP1_PWM_Deinitialize.
void(* Enable )(void)
Pointer to SCCPx_PWM_Enable e.g. SCCP1_PWM_Enable.
void(* Disable )(void)
Pointer to SCCPx_PWM_Disable e.g. SCCP1_PWM_Disable.
void(* PeriodSet )(size_t periodCount)
Pointer to SCCPx_PWM_PeriodSet e.g. SCCP1_PWM_PeriodSet.
void(* DutyCycleSet )(size_t dutyCycleCount)
Pointer to SCCPx_PWM_DutyCycleSet e.g. SCCP1_PWM_DutyCycleSet.
void(* DeadTimeSet )(size_t deadTimeCount)
Pointer to SCCPx_PWM_DeadTimeSet e.g. SCCP1_PWM_DeadTimeSet (This feature is hardware dependent)
void(* OutputModeSet )(enum PWM_OUTPUT_MODES outputMode)
Pointer to SCCPx_PWM_OutputModeSet e.g. SCCP1_PWM_OutputModeSet (This feature is hardware dependent)
void(* SoftwareTriggerSet )(void)
Pointer to SCCPx_PWM_SoftwareTriggerSet e.g. SCCP1_PWM_SoftwareTriggerSet.
void(* CallbackRegister )(void(*handler)(void))
Pointer to SCCPx_PWM_CallbackRegister e.g. SCCP1_PWM_CallbackRegister.
void(* Tasks )(void)
Pointer to SCCPx_PWM_Tasks e.g. SCCP1_PWM_Tasks (Supported only in polling mode)
3.16.2.1.2 Field Documentation
CallbackRegister
void(* CallbackRegister) (void(*handler)(void))
Pointer to SCCPx_PWM_CallbackRegister e.g. SCCP1_PWM_CallbackRegister.
DeadTimeSet
void(* DeadTimeSet) (size_t deadTimeCount)
Pointer to SCCPx_PWM_DeadTimeSet e.g. SCCP1_PWM_DeadTimeSet (This feature is hardware dependent)
Deinitialize
void(* Deinitialize) (void)
Pointer to SCCPx_PWM_Deinitialize e.g. SCCP1_PWM_Deinitialize.
Disable
void(* Disable) (void)
Pointer to SCCPx_PWM_Disable e.g. SCCP1_PWM_Disable.
DutyCycleSet
void(* DutyCycleSet) (size_t dutyCycleCount)
Pointer to SCCPx_PWM_DutyCycleSet e.g. SCCP1_PWM_DutyCycleSet.
Enable
void(* Enable) (void)
Pointer to SCCPx_PWM_Enable e.g. SCCP1_PWM_Enable.
Initialize
void(* Initialize) (void)
Pointer to SCCPx_PWM_Initialize e.g. SCCP1_PWM_Initialize.
OutputModeSet
void(* OutputModeSet) (enum PWM_OUTPUT_MODES outputMode)
Pointer to SCCPx_PWM_OutputModeSet e.g. SCCP1_PWM_OutputModeSet (This feature is hardware dependent)
PeriodSet
void(* PeriodSet) (size_t periodCount)
Pointer to SCCPx_PWM_PeriodSet e.g. SCCP1_PWM_PeriodSet.
SoftwareTriggerSet
void(* SoftwareTriggerSet) (void)
Pointer to SCCPx_PWM_SoftwareTriggerSet e.g. SCCP1_PWM_SoftwareTriggerSet.
Tasks
void(* Tasks) (void)
Pointer to SCCPx_PWM_Tasks e.g. SCCP1_PWM_Tasks (Supported only in polling mode)
3.16.3 File Documentation
3.16.3.1 source/pwm_interface.h File Reference
#include <stddef.h> #include "pwm_types.h"
3.16.3.1.1 Data structures
struct PWM_INTERFACE
Structure containing the function pointers of PWM generator driver.
3.16.3.1.2 Detailed Description
PWM Generated Driver Interface Header File
3.16.3.2 source/pwm_types.h File Reference
This is the generated driver types header file for the PWM driver.
3.16.3.2.1 Enumerations
enum PWM_OUTPUT_MODES { OUTPUT_MODES_NOT_AVAILABLE }
Enumeration containing the output modes of PWM driver.
3.16.3.2.2 Detailed Description
This is the generated driver types header file for the PWM driver.
PWM Generated Driver Types Header File
3.16.3.3 source/sccp1.h File Reference
This is the generated driver header file for the SCCP1 driver.
#include <stddef.h> #include "pwm_interface.h"
3.16.3.3.1 Functions
void SCCP1_PWM_Initialize (void)
Initializes the SCCP1 Pulse driver. This function must be called before any other SCCP1 function is called.
void SCCP1_PWM_Deinitialize (void)
Deinitializes the SCCP1 to POR values.
void SCCP1_PWM_Enable (void)
Enables the SCCP1 module.
void SCCP1_PWM_Disable (void)
Disables the SCCP1 module.
void SCCP1_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
void SCCP1_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
void SCCP1_PWM_SoftwareTriggerSet (void)
This function sets the manual trigger.
void SCCP1_PWM_CallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for SCCP1 PWM event.
void SCCP1_PWM_Callback (void)
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using SCCP1_PWM_CallbackRegister.
void SCCP1_PWM_Tasks (void)
This function is used to implement the tasks for polled implementations.
3.16.3.3.2 Macros
#define PWM1_Initialize SCCP1_PWM_Initialize
This macro defines the Custom Name for SCCP1_PWM_Initialize API.
#define PWM1_Deinitialize SCCP1_PWM_Deinitialize
This macro defines the Custom Name for SCCP1_PWM_Deinitialize API.
#define PWM1_Enable SCCP1_PWM_Enable
This macro defines the Custom Name for SCCP1_PWM_Enable API.
#define PWM1_Disable SCCP1_PWM_Disable
This macro defines the Custom Name for SCCP1_PWM_Disable API.
#define PWM1_PeriodSet SCCP1_PWM_PeriodSet
This macro defines the Custom Name for SCCP1_PWM_PeriodSet API.
#define PWM1_DutyCycleSet SCCP1_PWM_DutyCycleSet
This macro defines the Custom Name for SCCP1_PWM_DutyCycleSet API.
#define PWM1_SoftwareTriggerSet SCCP1_PWM_SoftwareTriggerSet
This macro defines the Custom Name for SCCP1_PWM_SoftwareTriggerSet API.
#define PWM1_Tasks SCCP1_PWM_Tasks
This macro defines the Custom Name for SCCP1_PWM_Tasks API.
#define PWM1_PWM_CallbackRegister SCCP1_PWM_CallbackRegister
This macro defines the Custom Name for SCCP1_PWM_CallbackRegister API.
3.16.3.3.3 Variables
const struct PWM_INTERFACE PWM1
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface. The default name e.g. PWM1 can be changed by the user in the MCCP user interface. This allows defining a structure with application specific name using the 'Custom Name' field. Application specific name allows the API Portability.
3.16.3.3.4 Detailed Description
This is the generated driver header file for the SCCP1 driver.
SCCP1 Generated Driver Header File