1.1.3 GENERIC AUDIO DRIVER CODEC Library
This topic describes the Generic Codec Driver Library.
Introduction
This library provides an Applications Programming Interface (API) to manage the Generic Codec that is serially interfaced to the I2C and I2S peripherals of a Microchip microcontroller for the purpose of providing audio solutions.
Description
This file contains the implementation of the Generic Codec driver, which provides a simple interface to manage a codec that can be interfaced to Microchip microcontroller. The user will need to modify it to match the requirements of their codec. Areas where code needs to be added or changed are marked with TO-DO!!
Note: this module assumes the codec is controlled over an I2C interface. The I2C Driver will need to be enabled in the MHC Project Graph. If another type of interface is used, the user will need to modify the code to suit. This module makes use of SYS_TIME. It will need to be enabled in the Project Graph.
This module assumes the an I2S interface is used for audio data output (to headphones or line-out) and input (from microphone or line-in).
The Generic Codec can be configured as either an I2S clock client (receives all clocks from the host), or I2S clock host (generates I2S clocks from a host clock input MCLK).
A typical interface of Generic Codec to a Microchip microcontroller using an I2C and SSC interface (configured as I2S), with the Generic Codec set up as the I2S clock client, is provided in the following diagram:
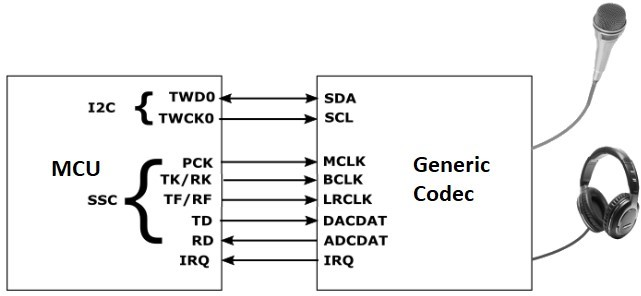
Using the Library
This topic describes the basic architecture of the Generic Codec Driver Library and provides information and examples on its use.
Interface Header File: drv_Generic.h
The interface to the Generic Codec Driver library is defined in the audio/driver/codec/Generic/drv_Generic.h header file. Any C language source (.c) file that uses the Generic Codec Driver library should include this header.
Library Source Files:
The Generic Codec Driver library source files are provided in the audio/driver/codec/Generic/src directory. This folder may contain optional files and alternate implementations. Please refer to Configuring the Library for instructions on how to select optional features and to Building the Library for instructions on how to build the library.
Example Applications:
This codec is not used directly by any demonstration applications. However the following applications could be looked at, to see how a codec such as the WM8904 or AK4954 is used:
audio/apps/audio_tone
audio/apps/microphone_loopback
Abstraction Model
This library provides a low-level abstraction of the Generic Codec Driver Library on the Microchip family microcontrollers with a convenient C language interface. This topic describes how that abstraction is modeled in software and introduces the library's interface.
Description
The abstraction model shown in the following diagram depicts how the Generic Codec Driver is positioned in the MPLAB Harmony framework. The Generic Codec Driver uses the I2C and I2S drivers for control and audio data transfers to the Generic module.
Generic Driver Abstraction Model
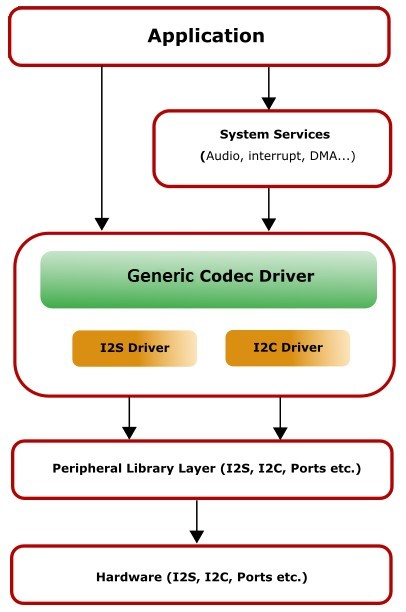
Library Overview
Refer to the Driver Library Overview section for information on how the driver operates in a system.
The Generic Codec Driver Library provides an API interface to transfer control commands and digital audio data to the serially interfaced Generic Codec module. The library interface routines are divided into various sub-sections, which address one of the blocks or the overall operation of the Generic Codec Driver Library.
Library Interface Section | Description |
---|---|
System Functions | Provides system module interfaces, device initialization, deinitialization, reinitialization, tasks and status functions. |
Client Setup Functions | Provides open and close functions. |
Data Transfer Functions | Provides data transfer functions, such as Buffer Read and Write. |
Settings Functions | Provides driver specific functions for settings, such as volume control and sampling rate. |
Other Functions | Miscellaneous functions, such as getting the driver’s version number and syncing to the LRCLK signal. |
Data Types and Constants | These data types and constants are required while interacting and setting up the Generic Codec Driver Library. |

How the Library Works
How the Library Works
The library provides interfaces to support:
System Functionality
Client Functionality
Setup (Initialization)
This topic describes system initialization, implementations, and includes a system access code example.
System Initialization
The system performs the initialization of the device driver with settings that affect only the instance of the device that is being initialized. During system initialization in the system_init.c file, each instance of the Generic module would be initialized with the following configuration settings (either passed dynamically at run time using DRV_Generic_INIT or by using Initialization Overrides) that are supported by the specific Generic device hardware:
Device requested power state: one of the System Module Power States. For specific details please refer to Data Types and Constants in the Library Interface section.
I2C driver module index. The module index should be same as the one used in initializing the I2C Driver
I2S driver module index. The module index should be same as the one used in initializing the I2S Driver
Sampling rate
Volume
Audio data format. The audio data format should match with the audio data format settings done in I2S driver initialization
Determines whether or not the microphone input is enabled
The DRV_Generic_Initialize API returns an object handle of the type SYS_MODULE_OBJ. The object handle returned by the Initialize interface would be used by the other system interfaces such as DRV_ Generic_Deinitialize, DRV_ Generic_Status and DRV_I2S_Tasks.
Client Access
This topic describes driver initialization and provides a code example.
Description
For the application to start using an instance of the module, it must call the DRV_Generic_Open function. The DRV_Generic_Open function provides a driver handle to the Generic Codec Driver instance for operations. If the driver is deinitialized using the function DRV_Generic_Deinitialize, the application must call the DRV_Generic_Open function again to set up the instance of the driver.
For the various options available for IO_INTENT, please refer to Data Types and Constants in the Library Interface section.

Example:
DRV_HANDLE handle; SYS_STATUS genericStatus; genericStatus Status = DRV_Generic_Status(sysObjects.genericStatus DevObject); if (SYS_STATUS_READY == genericStatus) { // The driver can now be opened. appData.genericClient.handle = DRV_Generic_Open (DRV_Generic_INDEX_0, DRV_IO_INTENT_WRITE | DRV_IO_INTENT_EXCLUSIVE); if(appData.genericClient.handle != DRV_HANDLE_INVALID) { appData.state = APP_STATE_Generic_SET_BUFFER_HANDLER; } else { SYS_DEBUG(0, "Find out what's wrong \r\n"); } } else { /* Generic Driver Is not ready */ }
Client Operations
This topic provides information on client operations.
Description
Client operations provide the API interface for control command and audio data transfer to the Generic Codec.
The following Generic Codec specific control command functions are provided:
DRV_Generic_SamplingRateSet
DRV_Generic_SamplingRateGet
DRV_Generic_VolumeSet
DRV_Generic_VolumeGet
DRV_Generic_MuteOn
DRV_Generic_MuteOff
These functions schedule a non-blocking control command transfer operation. These functions submit the control command request to the Generic Codec. These functions submit the control command request to I2C Driver transmit queue, the request is processed immediately if it is the first request, or processed when the previous request is complete.
DRV_Generic_BufferAddWrite, DRV_Generic_BufferAddRead, and DRV_Generic_BufferAddWriteRead are buffered data operation functions. These functions schedule non-blocking audio data transfer operations. These functions add the request to I2S Driver transmit or receive buffer queue depends on the request type, and are executed immediately if it is the first buffer, or executed later when the previous buffer is complete. The driver notifies the client with DRV_Generic_BUFFER_EVENT_COMPLETE, DRV_Generic_BUFFER_EVENT_ERROR, or DRV_Generic_BUFFER_EVENT_ABORT events.

Configuring the Library
The configuration of the I2S Driver Library is based on the file configurations.h, as generated by the MHC.
This header file contains the configuration selection for the I2S Driver Library. Based on the selections made, the I2S Driver Library may support the selected features. These configuration settings will apply to all instances of the I2S Driver Library.
This header can be placed anywhere; however, the path of this header needs to be present in the include search path for a successful build. Refer to the Applications Help section for more details.
System Configuration
Macros
Name | Description |
---|---|
DRV_GENERICCODEC_AUDIO_DATA_FORMAT_MACRO | Specifies the audio data format for the codec. |
DRV_GENERICCODEC_AUDIO_SAMPLING_RATE | Specifies the initial baud rate for the codec. |
DRV_GENERICCODEC_CLIENTS_NUMBER | Sets up the maximum number of clients that can be connected to any hardware instance. |
DRV_GENERICCODEC_I2C_DRIVER_MODULE_INDEX_IDXx | Specifies the instance number of the I2C interface. |
DRV_GENERICCODEC_I2S_DRIVER_MODULE_INDEX_IDXx | Specifies the instance number of the I2S interface. |
DRV_GENERICCODEC_INSTANCES_NUMBER | Sets up the maximum number of hardware instances that can be supported. |
DRV_GENERICCODEC_VOLUME | Specifies the initial volume level. |