2.4 CMP DAC Driver
Overview
The high-speed analog comparator module provides a method to monitor voltage, current and other critical signals in a power conversion application that may be too fast for the CPU and ADC to capture.
The high-speed analog comparator module is comprised of a high-speed comparator, Pulse Density Modulation (PDM) DAC and a slope compensation unit. The slope compensation unit provides a user-defined slope which can be used to alter the DAC output. This feature is useful in applications, such as Peak Current mode control, where slope compensation is required to maintain the stability of the power supply
Features
- The user can configure the DAC Mode based on their application needs.
- There are four modes supported : DC-Mode, Slope Mode, Hysteretic Mode and Triangle Wave Mode.
- The Clock and Divider settings can be configured in the PLIB dependency of the driver module.
DAC Modes
DC-Mode
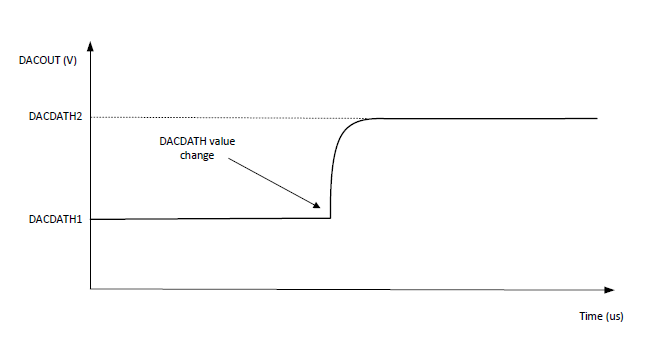
Slope Mode
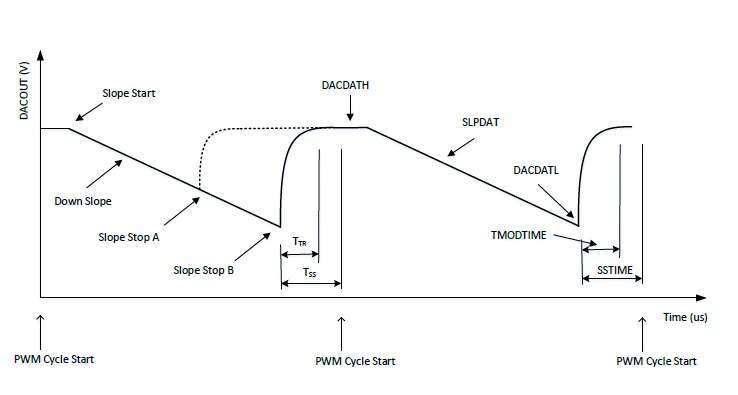
Hysteretic Mode
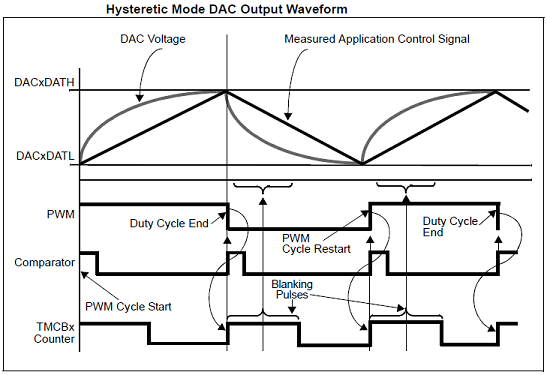
Triangle Wave Mode
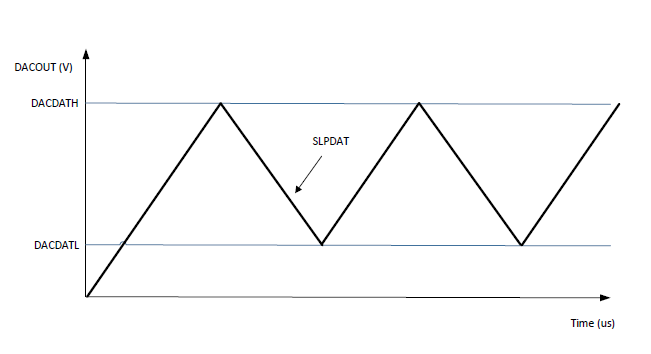
2.4.1 Module Documentation
2.4.1.1 CMP DAC Driver
High Speed Analog Comparator with Slope Compensation DAC driver using dsPIC MCUs.
2.4.1.1.1 Module description
High Speed Analog Comparator with Slope Compensation DAC driver using dsPIC MCUs.
Data structures
struct DAC_DC_INTERFACE
Structure containing the function pointers of DAC driver in DC mode.
struct CMP_INTERFACE
Structure containing the function pointers of CMP driver.
Functions
void CMP1_Initialize (void)
Initialize the CMP1 module.
void CMP1_Deinitialize (void)
Deinitializes the CMP1 to POR values.
bool CMP1_StatusGet (void)
Returns the comparator output status.
void CMP1_Enable (void)
Enables the common DAC module.
void CMP1_Disable (void)
Disables the common DAC module.
void CMP1_DACEnable (void)
Enables the individual DAC module.
void CMP1_DACDisable (void)
Disables the individual DAC module.
void CMP1_DACDataWrite (size_t value)
CMP DAC Data write to register.
void CMP1_EventCallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for CMP1 Event event.
void CMP1_EventCallback (void)
This is the default callback with weak attribute.
void CMP1_Tasks (void)
The Task function can be called in the main application using the High Speed.
Variables
const struct CMP_INTERFACE CMP_DAC1
Structure object of type CMP_INTERFACE with the custom name given by the user in the Melody Driver User interface.
2.4.1.1.2 Function Documentation
CMP1_DACDataWrite()
void CMP1_DACDataWrite (size_t value)
CMP DAC Data write to register.
in | value |
- DAC Data write value |
none |
CMP1_DACDisable()
void CMP1_DACDisable (void )
Disables the individual DAC module.
none |
none |
CMP1_DACEnable()
void CMP1_DACEnable (void )
Enables the individual DAC module.
none |
none |
CMP1_Deinitialize()
void CMP1_Deinitialize (void )
Deinitializes the CMP1 to POR values.
none |
none |
CMP1_Disable()
void CMP1_Disable (void )
Disables the common DAC module.
none |
none |
CMP1_Enable()
void CMP1_Enable (void )
Enables the common DAC module.
none |
none |
CMP1_EventCallback()
void CMP1_EventCallback (void )
This is the default callback with weak attribute.
The user can override and implement the default callback without weak attribute or can register a custom callback function using
CMP1_EventCallbackRegister.
none |
none |
CMP1_EventCallbackRegister()
void CMP1_EventCallbackRegister (void(*)(void) handler)
This function can be used to override default callback and to define custom callback for CMP1 Event event.
in | handler |
- Address of the callback function. |
none |
CMP1_Initialize()
void CMP1_Initialize (void )
Initialize the CMP1 module.
none |
none |
CMP1_StatusGet()
bool CMP1_StatusGet (void )
Returns the comparator output status.
none |
true - Comparator output is high false - Comparator output is low |
CMP1_Tasks()
void CMP1_Tasks (void )
The Task function can be called in the main application using the High Speed.
Comparator, when interrupts are not used. This would thus introduce the polling mode feature of the Analog Comparator.
none |
none |
2.4.1.1.3 Variable Documentation
CMP_DAC1
const struct CMP_INTERFACE CMP_DAC1
Structure object of type CMP_INTERFACE with the custom name given by the user in the Melody Driver User interface.
The default name e.g. CMP_DAC1 can be changed by the user in the CMP user interface. This allows defining a structure with application specific name using the 'Custom Name' field. Application specific name allows the API Portability.
2.4.2 Class Documentation
2.4.2.1 CMP_INTERFACE Struct Reference
Structure containing the function pointers of CMP driver.
2.4.2.1.1 Detailed Description
Structure containing the function pointers of CMP driver.
#include <cmp_interface.h>
Public Attributes
void(* Initialize )(void)
Pointer to CMPx_Initialize e.g. CMP1_Initialize.
void(* Deinitialize )(void)
Pointer to CMPx_Deinitialize e.g. CMP1_Deinitialize.
void(* Enable )(void)
Pointer to CMPx_Enable e.g. CMP1_Enable.
void(* Disable )(void)
Pointer to CMPx_Disable e.g. CMP1_Disable.
bool(* StatusGet )(void)
Pointer to CMPx_StatusGet e.g. CMP1_StatusGet.
void(* EventCallbackRegister )(void(*handler)(void))
Pointer to CMPx_EventCallbackRegister e.g. CMP1_EventCallbackRegister.
void(* Tasks )(void)
Pointer to CMPx_Tasks e.g. CMP1_Tasks (Supported only in polling mode)
const struct DAC_DC_INTERFACE * cmp_dac_dc_interface
Pointer to DAC_DC_INTERFACE.
2.4.2.1.2 Member Data Documentation
cmp_dac_dc_interface
const struct DAC_DC_INTERFACE* cmp_dac_dc_interface
Pointer to DAC_DC_INTERFACE.
Deinitialize
void(* Deinitialize) (void)
Pointer to CMPx_Deinitialize e.g. CMP1_Deinitialize.
Disable
void(* Disable) (void)
Pointer to CMPx_Disable e.g. CMP1_Disable.
Enable
void(* Enable) (void)
Pointer to CMPx_Enable e.g. CMP1_Enable.
EventCallbackRegister
void(* EventCallbackRegister) (void(*handler)(void))
Pointer to CMPx_EventCallbackRegister e.g. CMP1_EventCallbackRegister.
Initialize
void(* Initialize) (void)
Pointer to CMPx_Initialize e.g. CMP1_Initialize.
StatusGet
bool(* StatusGet) (void)
Pointer to CMPx_StatusGet e.g. CMP1_StatusGet.
Tasks
void(* Tasks) (void)
Pointer to CMPx_Tasks e.g. CMP1_Tasks (Supported only in polling mode)
2.4.2.2 DAC_DC_INTERFACE Struct Reference
Structure containing the function pointers of DAC driver in DC mode.
2.4.2.2.1 Detailed Description
Structure containing the function pointers of DAC driver in DC mode.
#include <cmp_interface.h>
Public Attributes
void(* Enable )(void)
Pointer to CMPx_DACEnable e.g. CMP1_DACEnable.
void(* Disable )(void)
Pointer to CMPx_DACDisable e.g. CMP1_DACDisable.
void(* DataWrite )(size_t value)
Pointer to CMPx_DACDataWrite e.g. CMP1_DACDataWrite.
2.4.2.2.2 Member Data Documentation
DataWrite
void(* DataWrite) (size_t value)
Pointer to CMPx_DACDataWrite e.g. CMP1_DACDataWrite.
Disable
void(* Disable) (void)
Pointer to CMPx_DACDisable e.g. CMP1_DACDisable.
Enable
void(* Enable) (void)
Pointer to CMPx_DACEnable e.g. CMP1_DACEnable.
2.4.3 File Documentation
2.4.3.1 source/cmp1.h File Reference
This is the generated driver header file for the CMP1 driver.
#include <stddef.h> #include <stdbool.h> #include "cmp_interface.h"
2.4.3.1.1 Functions
void CMP1_Initialize (void)
Initialize the CMP1 module.
void CMP1_Deinitialize (void)
Deinitializes the CMP1 to POR values.
bool CMP1_StatusGet (void)
Returns the comparator output status.
void CMP1_Enable (void)
Enables the common DAC module.
void CMP1_Disable (void)
Disables the common DAC module.
void CMP1_DACEnable (void)
Enables the individual DAC module.
void CMP1_DACDisable (void)
Disables the individual DAC module.
void CMP1_DACDataWrite (size_t value)
CMP DAC Data write to register.
void CMP1_EventCallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for CMP1 Event event.
void CMP1_EventCallback (void)
This is the default callback with weak attribute.
void CMP1_Tasks (void)
The Task function can be called in the main application using the High Speed.
2.4.3.1.2 Variables
const struct CMP_INTERFACE CMP_DAC1
Structure object of type CMP_INTERFACE with the custom name given by the user in the Melody Driver User interface.
2.4.3.1.3 Detailed Description
This is the generated driver header file for the CMP1 driver.
CMP1 Generated Driver Header File
2.4.3.2 source/cmp_interface.h File Reference
#include <stdbool.h> #include <stddef.h>
2.4.3.2.1 Data structures
struct DAC_DC_INTERFACE
Structure containing the function pointers of DAC driver in DC mode.
struct CMP_INTERFACE
Structure containing the function pointers of CMP driver.
2.4.3.2.2 Detailed Description
CMP Generated Driver Interface Header File