2.20 PWM Driver
Overview
PWM is used to generate the PWM pulses, this PWM is used to drive motor and other applications.
Features
- PWM Frequency and the Duty cycle is user configurable, the range of the Frequency value depends on the clock source selected from the Hardware Dependency module (PLIB).
- Internal timer operates in Synchronization (Sync) or Trigger mode.
- Sync sources are user configurable, the timer Reset or clear occurs when the input selected by the Sync source is asserted. The Timer immediately begins to count again from zero unless it is held for some other reason.
- In Trigger operation, the timer is held in Reset until the input selected by Sync source is asserted.
The following waveform represents the PWM output and the effect of Sync source.
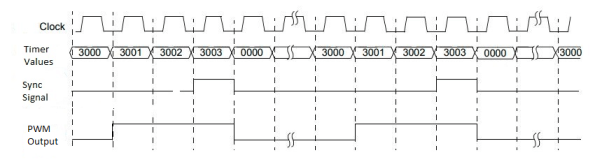
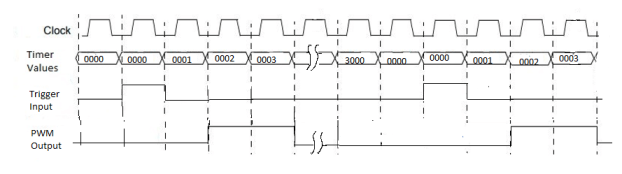
2.20.1 Module Documentation
2.20.1.1 PWM Driver
Pulse Width Modulation Driver using dsPIC MCUs.
2.20.1.1.1 Module description
Pulse Width Modulation Driver using dsPIC MCUs.
Data structures
struct PWM_INTERFACE
Structure containing the function pointers of PWM generator driver.
Enumerations
enum PWM_OUTPUT_MODES { OUTPUT_SCAN_MODE = 0x6, BRUSH_DC_OUTPUT_FORWARD = 0x5, BRUSH_DC_OUTPUT_REVERSE = 0x4, HALF_BRIDGE_OUTPUT = 0x2, PUSH_PULL_OUTPUT = 0x1, STEERABLE_SINGLE_OUTPUT = 0x0 }
Enumeration containing the output modes of PWM driver.
Functions
void MCCP9_PWM_Initialize (void)
Initializes the MCCP9 Pulse driver. This function must be called before any other MCCP9 function is called.
void MCCP9_PWM_Deinitialize (void)
Deinitializes the MCCP9 to POR values.
void MCCP9_PWM_Enable (void)
Enables the MCCP9 module.
void MCCP9_PWM_Disable (void)
Disables the MCCP9 module.
void MCCP9_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
void MCCP9_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
void MCCP9_PWM_DeadTimeSet (size_t deadTimeCount)
Sets the dead time.
void MCCP9_PWM_OutputModeSet (enum PWM_OUTPUT_MODES outputMode)
Sets output mode of MCCP.
void MCCP9_PWM_SoftwareTriggerSet (void)
This function sets the manual trigger.
void MCCP9_PWM_CallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for MCCP9 PWM event.
void MCCP9_PWM_Callback (void)
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using MCCP9_PWM_CallbackRegister.
void MCCP9_PWM_Tasks (void)
This function is used to implement the tasks for polled implementations.
Variables
const struct PWM_INTERFACE PWM9
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface.
2.20.1.1.2 Function Documentation
MCCP9_PWM_Callback()
void MCCP9_PWM_Callback (void )
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using MCCP9_PWM_CallbackRegister.
none |
none |
MCCP9_PWM_CallbackRegister()
void MCCP9_PWM_CallbackRegister (void(*)(void) handler)
This function can be used to override default callback and to define custom callback for MCCP9 PWM event.
in | handler |
- Address of the callback function |
none |
MCCP9_PWM_DeadTimeSet()
void MCCP9_PWM_DeadTimeSet (size_t deadTimeCount)
Sets the dead time.
in | deadTimeCount |
- number of cycles of wait time |
none |
MCCP9_PWM_Deinitialize()
void MCCP9_PWM_Deinitialize (void )
Deinitializes the MCCP9 to POR values.
none |
none |
MCCP9_PWM_Disable()
void MCCP9_PWM_Disable (void )
Disables the MCCP9 module.
none |
none |
MCCP9_PWM_DutyCycleSet()
void MCCP9_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
in | dutyCycleCount |
- number of cycles of ON time |
none |
MCCP9_PWM_Enable()
void MCCP9_PWM_Enable (void )
Enables the MCCP9 module.
none |
none |
MCCP9_PWM_Initialize()
void MCCP9_PWM_Initialize (void )
Initializes the MCCP9 Pulse driver. This function must be called before any other MCCP9 function is called.
none |
none |
MCCP9_PWM_OutputModeSet()
void MCCP9_PWM_OutputModeSet (enum PWM_OUTPUT_MODES outputMode)
Sets output mode of MCCP.
in | outputMode |
- mode of operation |
none |
MCCP9_PWM_PeriodSet()
void MCCP9_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
in | periodCount |
- number of clock counts for PWM Period |
none |
MCCP9_PWM_SoftwareTriggerSet()
void MCCP9_PWM_SoftwareTriggerSet (void )
This function sets the manual trigger.
none |
none |
MCCP9_PWM_Tasks()
void MCCP9_PWM_Tasks (void )
This function is used to implement the tasks for polled implementations.
none |
none |
2.20.1.1.3 Enumeration Type Documentation
PWM_OUTPUT_MODES
enum PWM_OUTPUT_MODES
Enumeration containing the output modes of PWM driver.
OUTPUT_SCAN_MODE |
Output Scan Mode |
BRUSH_DC_OUTPUT_FORWARD |
Brush DC Output Forward Mode |
BRUSH_DC_OUTPUT_REVERSE |
Brush DC Output Reverse Mode |
HALF_BRIDGE_OUTPUT |
Half Bridge Output Mode |
PUSH_PULL_OUTPUT |
Push Pull Output Mode |
STEERABLE_SINGLE_OUTPUT |
Steerable Single Output Mode |
2.20.1.1.4 Variable Documentation
PWM9
const struct PWM_INTERFACE PWM9
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface.
The default name e.g. PWM1 can be changed by the user in the MCCP user interface. This allows defining a structure with application specific name using the 'Custom Name' field. Application specific name allows the API Portability.
2.20.2 Class Documentation
2.20.2.1 PWM_INTERFACE Struct Reference
Structure containing the function pointers of PWM generator driver.
2.20.2.1.1 Detailed Description
Structure containing the function pointers of PWM generator driver.
#include <pwm_interface.h>
Public Attributes
void(* Initialize )(void)
Pointer to SCCPx_PWM_Initialize e.g. SCCP1_PWM_Initialize.
void(* Deinitialize )(void)
Pointer to SCCPx_PWM_Deinitialize e.g. SCCP1_PWM_Deinitialize.
void(* Enable )(void)
Pointer to SCCPx_PWM_Enable e.g. SCCP1_PWM_Enable.
void(* Disable )(void)
Pointer to SCCPx_PWM_Disable e.g. SCCP1_PWM_Disable.
void(* PeriodSet )(size_t periodCount)
Pointer to SCCPx_PWM_PeriodSet e.g. SCCP1_PWM_PeriodSet.
void(* DutyCycleSet )(size_t dutyCycleCount)
Pointer to SCCPx_PWM_DutyCycleSet e.g. SCCP1_PWM_DutyCycleSet.
void(* DeadTimeSet )(size_t deadTimeCount)
Pointer to SCCPx_PWM_DeadTimeSet e.g. SCCP1_PWM_DeadTimeSet (This feature is hardware dependent)
void(* OutputModeSet )(enum PWM_OUTPUT_MODES outputMode)
Pointer to SCCPx_PWM_OutputModeSet e.g. SCCP1_PWM_OutputModeSet (This feature is hardware dependent)
void(* SoftwareTriggerSet )(void)
Pointer to SCCPx_PWM_SoftwareTriggerSet e.g. SCCP1_PWM_SoftwareTriggerSet.
void(* CallbackRegister )(void(*handler)(void))
Pointer to SCCPx_PWM_CallbackRegister e.g. SCCP1_PWM_CallbackRegister.
void(* Tasks )(void)
Pointer to SCCPx_PWM_Tasks e.g. SCCP1_PWM_Tasks (Supported only in polling mode)
2.20.2.1.2 Member Data Documentation
CallbackRegister
void(* CallbackRegister) (void(*handler)(void))
Pointer to SCCPx_PWM_CallbackRegister e.g. SCCP1_PWM_CallbackRegister.
DeadTimeSet
void(* DeadTimeSet) (size_t deadTimeCount)
Pointer to SCCPx_PWM_DeadTimeSet e.g. SCCP1_PWM_DeadTimeSet (This feature is hardware dependent)
Deinitialize
void(* Deinitialize) (void)
Pointer to SCCPx_PWM_Deinitialize e.g. SCCP1_PWM_Deinitialize.
Disable
void(* Disable) (void)
Pointer to SCCPx_PWM_Disable e.g. SCCP1_PWM_Disable.
DutyCycleSet
void(* DutyCycleSet) (size_t dutyCycleCount)
Pointer to SCCPx_PWM_DutyCycleSet e.g. SCCP1_PWM_DutyCycleSet.
Enable
void(* Enable) (void)
Pointer to SCCPx_PWM_Enable e.g. SCCP1_PWM_Enable.
Initialize
void(* Initialize) (void)
Pointer to SCCPx_PWM_Initialize e.g. SCCP1_PWM_Initialize.
OutputModeSet
void(* OutputModeSet) (enum PWM_OUTPUT_MODES outputMode)
Pointer to SCCPx_PWM_OutputModeSet e.g. SCCP1_PWM_OutputModeSet (This feature is hardware dependent)
PeriodSet
void(* PeriodSet) (size_t periodCount)
Pointer to SCCPx_PWM_PeriodSet e.g. SCCP1_PWM_PeriodSet.
SoftwareTriggerSet
void(* SoftwareTriggerSet) (void)
Pointer to SCCPx_PWM_SoftwareTriggerSet e.g. SCCP1_PWM_SoftwareTriggerSet.
Tasks
void(* Tasks) (void)
Pointer to SCCPx_PWM_Tasks e.g. SCCP1_PWM_Tasks (Supported only in polling mode)
2.20.3 File Documentation
2.20.3.1 source/mccp9.h File Reference
This is the generated driver header file for the MCCP9 driver.
#include <stddef.h> #include "pwm_interface.h" #include "pwm_types.h"
2.20.3.1.1 Functions
void MCCP9_PWM_Initialize (void)
Initializes the MCCP9 Pulse driver. This function must be called before any other MCCP9 function is called.
void MCCP9_PWM_Deinitialize (void)
Deinitializes the MCCP9 to POR values.
void MCCP9_PWM_Enable (void)
Enables the MCCP9 module.
void MCCP9_PWM_Disable (void)
Disables the MCCP9 module.
void MCCP9_PWM_PeriodSet (size_t periodCount)
Sets the cycle width.
void MCCP9_PWM_DutyCycleSet (size_t dutyCycleCount)
Sets the ON pulse width.
void MCCP9_PWM_DeadTimeSet (size_t deadTimeCount)
Sets the dead time.
void MCCP9_PWM_OutputModeSet (enum PWM_OUTPUT_MODES outputMode)
Sets output mode of MCCP.
void MCCP9_PWM_SoftwareTriggerSet (void)
This function sets the manual trigger.
void MCCP9_PWM_CallbackRegister (void(*handler)(void))
This function can be used to override default callback and to define custom callback for MCCP9 PWM event.
void MCCP9_PWM_Callback (void)
This is the default callback with weak attribute. The user can override and implement the default callback without weak attribute or can register a custom callback function using MCCP9_PWM_CallbackRegister.
void MCCP9_PWM_Tasks (void)
This function is used to implement the tasks for polled implementations.
2.20.3.1.2 Variables
const struct PWM_INTERFACE PWM9
Structure object of type PWM_INTERFACE with the custom name given by the user in the Melody Driver User interface.
2.20.3.1.3 Detailed Description
This is the generated driver header file for the MCCP9 driver.
MCCP9 Generated Driver Header File
Firmware Driver Version 2.1.0 PLIB Version 1.5.0 |
2.20.3.2 source/pwm_interface.h File Reference
#include <stddef.h> #include "pwm_types.h"
2.20.3.2.1 Data structures
struct PWM_INTERFACE
Structure containing the function pointers of PWM generator driver.
2.20.3.2.2 Detailed Description
PWM Generated Driver Interface Header File
2.20.3.3 source/pwm_types.h File Reference
This is the generated driver types header file for the PWM driver.
2.20.3.3.1 Enumerations
enum PWM_OUTPUT_MODES { OUTPUT_SCAN_MODE = 0x6, BRUSH_DC_OUTPUT_FORWARD = 0x5, BRUSH_DC_OUTPUT_REVERSE = 0x4, HALF_BRIDGE_OUTPUT = 0x2, PUSH_PULL_OUTPUT = 0x1, STEERABLE_SINGLE_OUTPUT = 0x0 }
Enumeration containing the output modes of PWM driver.
2.20.3.3.2 Detailed Description
This is the generated driver types header file for the PWM driver.
PWM Generated Driver Types Header File