31.6.3 mips_iir16
Description
Computes a single-sample infinite impulse response (IIR) filter with coefficients specified in coeffs. The number of biquad sections composing the filter is given by the parameter B. The scale parameter specifies the amount of right shift applied to the input value of each biquad. Each biquad section is specified by four coefficients – A1, A2, B1, and B2 – and has two state variables stored inside delayline.°± The output of each biquad section becomes input to the next one. The output of the final section is returned as result of the mips_iir16() function.
The operations performed for each biquad section are illustrated below:
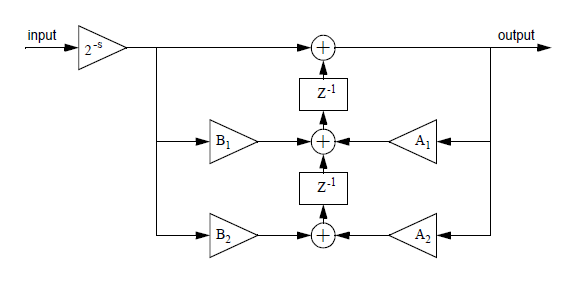
Include
dsplib_dsp.h
Prototype
int16
mips_iir16
(
int16 in,
int16 *coeffs,
int16 *delayline,
int B,
int scale
);
Argument
in: Input value in Q15 format.
coeffs: Array of 4B 16-bit fixed-point coefficients prepared by mips_iir16_setup().
delayline: Delay line array holding 2B state 16-bit state variables.
B: Number of biquad sections.
scale: Scaling factor: divide the input to each biquad by 2scale.
Return Value
IIR filter output value in fractional Q15 format.
Remarks
- The pointers coeffs and delayline must be aligned on a 4-byte boundary.
- B must be larger than or equal to 2 and a multiple of 2.
Notes
The coeffs array contains four coefficients for each biquad. The coefficients are conveniently specified in an array of biquad16 structures, which is converted to the appropriate internal representation by the mips_iir16_setup() function. All elements of the delayline array must be initialized to zero before the first call to mips_iir16(). Both delayline and coeffs have formats that are implementation-dependent and their contents should not be changed directly.
Example
int i;
int B = 4;
biquad16 bq[B];
int16 coeffs[4*B];
int16 delayline[2*B];
int16 indata, outdata;
for (i = 0; i < 2*B; i++)
delayline[i] = 0;
// load coefficients into bq here
...
mips_iir16_setup(coeffs, bq, B);
while (true)
{
// get input data value into indata
...
outdata = mips_iir16(indata, coeffs, delayline, B, 2);
// do something with outdata
...
}