1.2.1.13.1 Abstraction Model
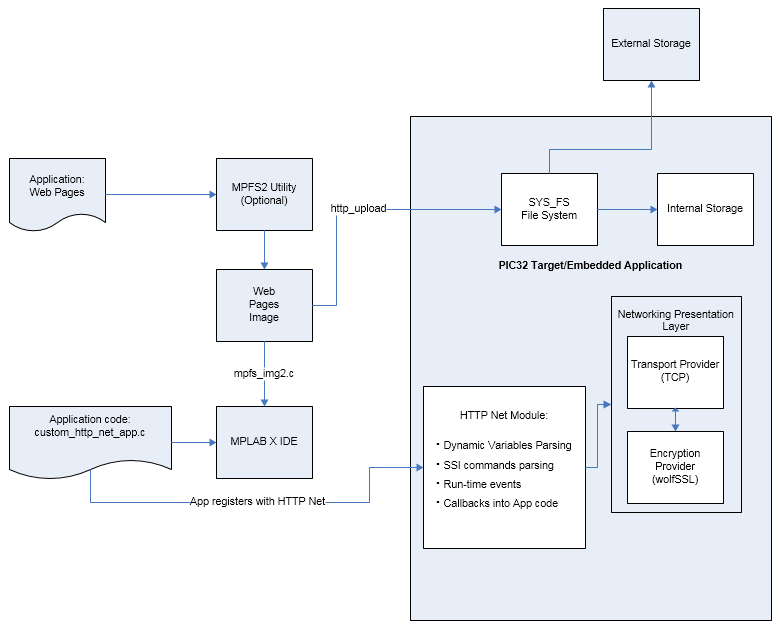
HTTP Net Features
The HTTP Net module is a HTTP server module that works using the Networking Presentation Layer of the MPLAB Harmony framework. This allows it to support both encrypted and plain text connections.
Some of the key features of the HTTP Net module include:
- The updated module implements HTTP 1.1 persistent connections by default, using the “Transfer-Encoding: chunked” HTTP header. It can be configured to operate with closing the connections like the previous HTTP server.
- The application has to dynamically register its HTTP processing functions with the HTTP Net server using the TCPIP_HTTP_NET_UserHandlerRegister call. The HTTP Net server no longer relies on well known function names.
- The HTTP Net server supports either secure or plain text connections, but not both
simultaneously. The specification of a secure or non-secure connection can be easily
done by:
- Selecting the server listening port: 80 or 443
- Using the module configuration flags
- The HTTP Net module reports run-time events that could be caught by the user application using the “eventReport” registered function that carries a TCPIP_HTTP_NET_EVENT_TYPE enumerated type
Dynamic Variables Processing
Parsing of the dynamic variables is done at run-time. The web pages could be changed at run-time, without the need for rebuilding the HTTP Net server. This allows the TCP/IP Stack to run as a library and only the application code to be changed accordingly. The application is required to register a “dynamicPrint” with the HTTP Net server. This function will be called at run-time to process the dynamic variables.
The supported syntax is ~var_name(param1, param2, …)~. When parsing a string like this within a web page, the HTTP Net server will invoke the “dynamicPrint” function using a TCPIP_HTTP_DYN_VAR_DCPT data structure that specifies the dynamic variable name, its number and type of parameters as well as each parameter value.
For example, the variable ~myVariable(2,6)~ will generate the “dynamicPrint” call with the following parameters:
varDcpt.dynName = "myVariable";
varDcpt.nArgs = 2;
varDcpt.dynArgs->argType = TCPIP_HTTP_DYN_ARG_TYPE_INT32;
varDcpt.dynArgs->argInt32 = 2;
(varDcpt.dynArgs + 1)->argType = TCPIP_HTTP_DYN_ARG_TYPE_INT32;
(varDcpt.dynArgs + 1)->argInt32 = 6;
String and int32_t variable types are currently supported.
The application needs to return a TCPIP_HTTP_DYN_PRINT_RES result specifying if it’s done with processing or it needs to be called again.
Applications no longer have direct access to the underlying transport socket for a HTTP connection. All of the data write operations need to go through the required API function: TCPIP_HTTP_NET_DynamicWrite. This function actually just attaches the data to be written to the connection, using data descriptors. The actual output occurs only when all data is gathered and the data size is known.
Buffers used in a TCPIP_HTTP_NET_DynamicWrite need to be persistent until the moment when the output is generated. RAM buffers are supported by using a “dynamicAck” (TCPIP_HTTP_NET_DynAcknowledge) type function. Once the output operation is completed the HTTP Net module will call back into the application indicating that the corresponding buffer is no longer used and can be freed/reused.
In situations where the dynamic variable print function needs to perform additional write operations, or simply needs to be called again, it must a special result code: TCPIP_HTTP_DYN_PRINT_RES_PROCESS_AGAIN/TCPIP_HTTP_DYN_PRINT_RES_AGAIN. Typically this is used when outputting large amounts of data that cannot fit into one single buffer write operation or when the data is not available all at once.
Include Files Processing
The HTTP Net module dynamically parses the web pages and the file inclusion is done by the module itself, completely transparent for the application.
The included files can contain dynamic variables that are parsed at run time.
File Processing
File processing consists of the following:
- An included file can include other files that have dynamic variables
- Application can include a file as part of its dynamic variable processing using the TCPIP_HTTP_NET_ConnectionFileInclude()API. That file can include other files.
- The only limit to the depth of inclusion is the memory resources and the TCPIP_HTTP_NET_MODULE_CONFIG:: maxRecurseLevel setting. A TCPIP_HTTP_NET_EVENT_DEPTH_ERROR event will be generated if the maximum depth is exceeded at run-time.
SSI Processing
At run-time, the HTTP Net server processes a subset of the Server Side Includes (SSI) commands. SSI is a portable way to add small amounts of dynamic content on web pages in a standard way that is supported by most HTTP servers. Currently the #include, #set and #echo commands are supported. Other commands will be eventually added. This allows a better compatibility with other existing HTTP servers and is targeted at the removal of proprietary extensions.
Whenever executing an SSI command within a web page, the HTTP Net server will notify the application by using the registered SSI callback: ssiNotify. This callback contains a TCPIP_HTTP_SSI_NOTIFY_DCPT pointer to a structure describing the SSI context needed for processing:
- File name the SSI command belongs to
- The SSI command line
- Number of SSI attributes and their values, etc.
The application can do any modifications it chooses to and returns a value instructing the HTTP Net server if the processing of the command is necessary or not.
SSI include Command
The SSI Include command allows the dynamic inclusion of a file. The supported syntax is:
<!--#include virtual="file_name" --> or <!--#include file="file_name" -->
Currently the arguments for both “virtual” and “file” commands are passed unaltered to the SYS_FS, so they behave identically. However it is recommended that the original SSI significance for these keywords should be maintained:
- Use “virtual” for specifying a URL relative to the document being server
- Use “file” for a file path, relative to the current directory (it cannot be an absolute path)
The ~inc:file_name~ keyword is maintained for backward compatibility. However the SSI include command should be preferred.
SSI set Command
The SSI set command allows to dynamically set a SSI variable value. The supported syntax is:
<!--#set var="v_name" value="v_value" -->
String or integer variables are supported. Variable reference is also supported:
<!--#set var="n_name" value="$otherVar" -->
This command will create or update the variable n_name to have the value of the variable otherVar, if it exists.
A new SSI variable will be created if a variable having the name v_name does not exist. If the variable v_name already exists, it will have its value updated as the result of this command.
An existing variable can be deleted using the empty value set command:
<!--#set var="v_name" value="" -->
SSI echo Command
The SSI echo command allows the dynamic print of a SSI variable. The supported syntax is:
<!--#echo var="v_name" -->
If the application ssiNotify exists, the HTTP Net server will call it and the application may choose to change the current value dynamically. If ssiNotify returns false, the HTTP Net server will display the current value of the variable v_name as part of the current page.
The SSI API can be used to evaluate or change the current value of the SSI variables:
- TCPIP_HTTP_NET_SSIVariableGet
- TCPIP_HTTP_NET_SSIVariableSet
- TCPIP_HTTP_NET_SSIVariableDelete
The maximum number of SSI variables is under the control of the application by using the configuration parameter: TCPIP_HTTP_NET_SSI_VARIABLES_NUMBER.
mpfs2.jar Utility
Dynamic Variable Processing
The HTTP Net server does not use the DynRecord.bin and FileRcrd.bin files for processing the dynamic variables. The parsing and processing of dynamic variables is done at run-time. Therefore, the mpfs2.jar utility is no longer necessary, other than to generate a mpfs_img2.c image file for the NVM MPFS image.
Application Custom Support Files
The application custom support files generated with MHC have new names: custom_http_net_app.c and http_net_print.c. However, the http_net_print.c file is not generated by the mpfs2 utility and is maintained only for easy comparison between HTTP and HTTP Net application processing. The custom_http_net_app.c file is entirely generated using a MHC template file and it is not dynamically updated in any way by the mpfs2 utility.
Generated File Name
Currently the name of the generated file for MPFS image is maintained unchanged: mpfs_img2.c.
MPFS Image Generation for Internal NVM Storage
The mpfs2 utility can still be used to generate the MPFS image for internal NVM storage. It can also be useful because it parses the web pages and comes out with the http_print.c file that contains the list of TCPIP_HTTP_Print functions. This can be helpful in gathering info about the dynamic variables that are contained within the web pages.