4 Low-Power Design Pattern
Low-power consumption may be an important application requirement. Here, we recommend an interrupt/callback or state machine-based design pattern.
Low Power is a critical element in many embedded applications, but especially important in battery-operated devices. Low-power techniques for microcontroller-based applications can be divided into two categories: those related to the microcontroller and its application, and those related to the overall embedded system design.
Power-Saving Techniques Related to the Microcontroller Itself and the Application It Runs
- Choose the Right
Microcontroller - Select a microcontroller designed for low-power
consumption. Almost all Microchip microcontrollers have power-saving modes like
sleep or deep sleep, which reduce power usage significantly when the device is
inactive.Tip: Newer families of Microchip microcontrollers tend to have more advanced low-power features, such as core independent peripherals, when enabling the MCU core to stay sleeping for longer.
- Effective Use of Power Modes - Utilize the different power modes of the microcontroller. For instance, use sleep mode during inactive periods and wake up the microcontroller using interrupts or timers only when needed.
- Minimize Active Time -
Reduce the active mode time of the microcontroller. Optimize your code to
complete tasks faster, allowing the microcontroller to return to a low-power
state sooner.Tip:
- Use Interrupts Over Polling - Use interrupt-driven programming instead of polling. Polling requires the microcontroller to be constantly active, whereas interrupts allow it to remain in a low-power state until a specific event occurs.
- Efficient Coding - Write efficient code that executes tasks quickly and effectively. Avoid unnecessary processing and loops that can keep the microcontroller in an Active state longer than necessary.
- Clock Management - Adjust the clock speed of the microcontroller. Running the microcontroller at a lower clock speed can significantly reduce power consumption. However, this needs to be balanced with the performance requirements of your application.
- Peripheral and GPIO
Management - Turn off unused peripherals. Most Microchip
microcontrollers allow disabling hardware components (like ADCs, timers and
communication interfaces) when not in use.Tip: Depending on the family of Microchip microcontrollers, different low-power strategies will be appropriate for unused GPIO pins.
Techniques Related to the Overall System Design
- Analog and Digital Component Selection - Choose low-power components for the system, including sensors, communication modules and other peripherals.
- Power Supply Optimization - Design an efficient power supply, considering voltage regulation and quiescent current.
- Power-efficient Communication Protocols - For systems involving communication, select energy-efficient protocols and manage the duty cycle of transmissions carefully.
- Temperature Management - Ensure the system operates efficiently across its intended temperature range, as higher temperatures can lead to increased power consumption.
- Hardware and Firmware Co-Design - Integrate hardware and firmware design to optimize overall power consumption, deciding which tasks are best handled by hardware and which by software.
4.1 Low-Power Considerations for GPIO
A key step in making any application low power, is setting all General Purpose Input/Output (GPIO) pins, to their lowest power mode. This may be different for used and unused pins. Here are some common strategies for handling unused GPIO pins to ensure low-power consumption:
- Input with Pull-up or Pull-down Resistors - Configuring unused GPIO pins as inputs with either pull-up or pull-down resistors can help reduce power consumption. This configuration prevents the pins from floating, which can cause higher power consumption due to sporadic switching and noise.
- Output Low or High State - Setting unused GPIO pins to a defined high or low output state can also be effective. This approach ensures that the pins are not floating and consuming unnecessary power. The choice between a high or low state depends on the specific circuitry and what results in lower power consumption.
- Analog Mode (if available) - Some microcontrollers allow setting GPIO pins to an analog mode, which can further reduce power consumption as it disconnects the pin from the digital input buffers.
- Disabling Schmitt Triggers - If the microcontroller has an option to disable Schmitt triggers on GPIO pins, doing so for unused pins can save power, as Schmitt triggers can increase power consumption due to their hysteresis characteristics.
- Disabling Internal Pull-up/Pull-down (if externally provided) - Disable the pull-up and pull-down resistors on the GPIO pins when using external pull-up or pull-down resistors for a more power-efficient result.
- Deep Sleep Modes: In some microcontrollers (when the device enters deep sleep modes), the GPIO pins state can automatically be configured to a low-power state, which might involve disabling digital input buffers or other power-consuming features.
4.2 MCC Melody Support for Low-Power
Support for Low Power is provided by System Drivers. This varies by the architecture of the supported microcontrollers. So, there are separate sections discussing how this is handled for AVR, PIC16F/18F and dsPIC.
4.2.1 MCC Melody Support for Low-Power in PIC16F/18F
The key system module for Low Power support in PIC16F/18F MCUs is the Peripheral Module Disable (PMD). The PMD provides the ability to selectively enable or disable a peripheral. The PMD puts the disabled peripherals in the lowest possible power state, thus reducing the overall power consumption of the microcontroller.
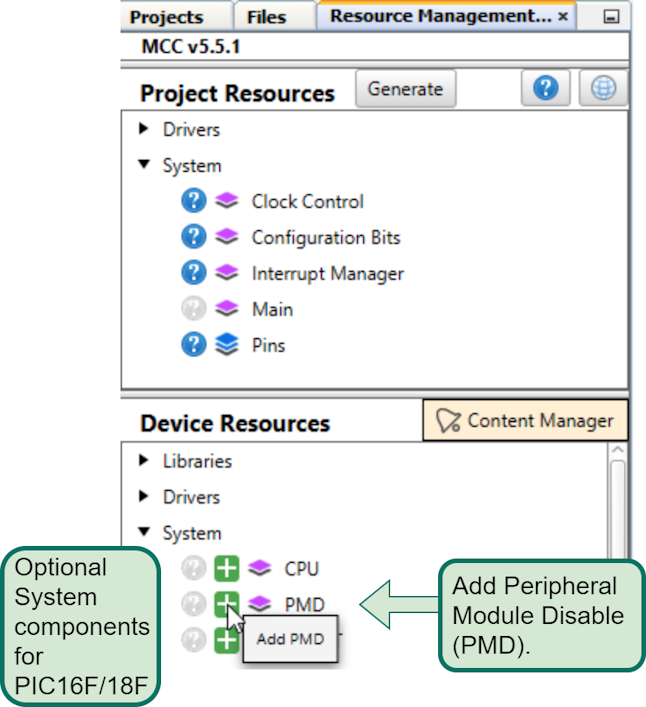
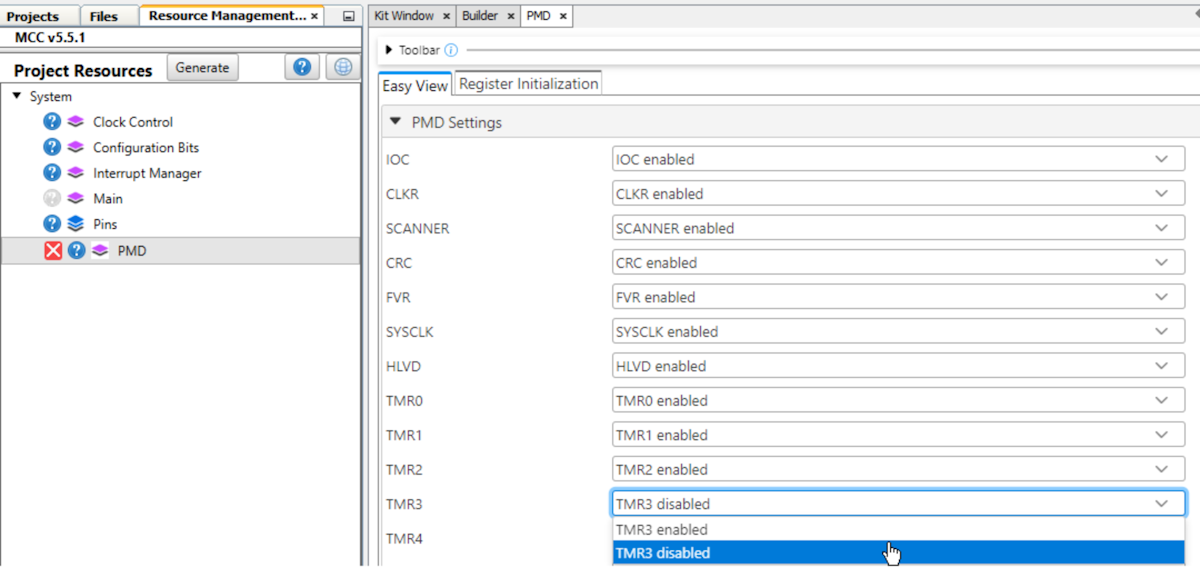
4.2.2 MCC Melody Support for Low-Power in AVR
The key system module for Low Power support in AVR MCUs is the Sleep Controller (SLPCTRL). SLPCTRL provides the ability to selectively put the AVR in various low power modes.
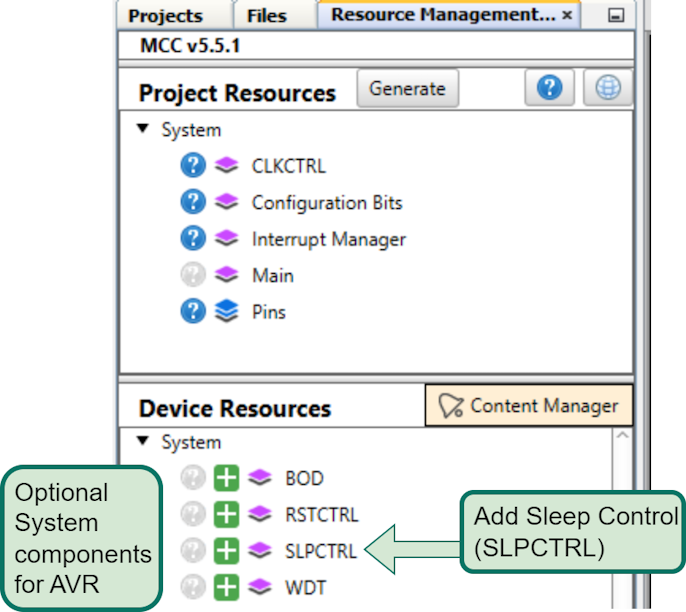
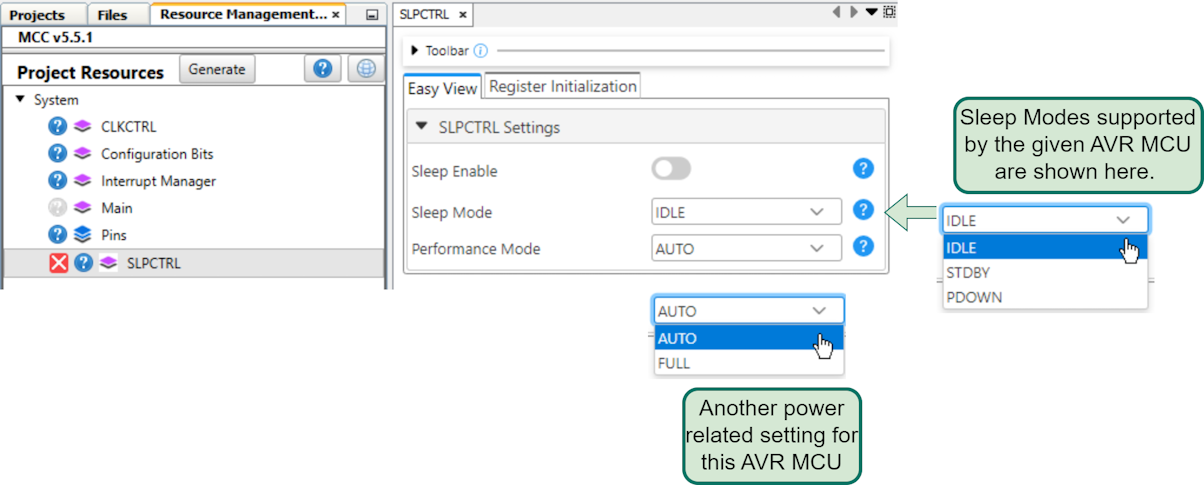
Sleep Control is very microcontroller specific. However, the Sleep Controller makes it easy to understand the features of the specific MCU family, by opening the relevant context help.
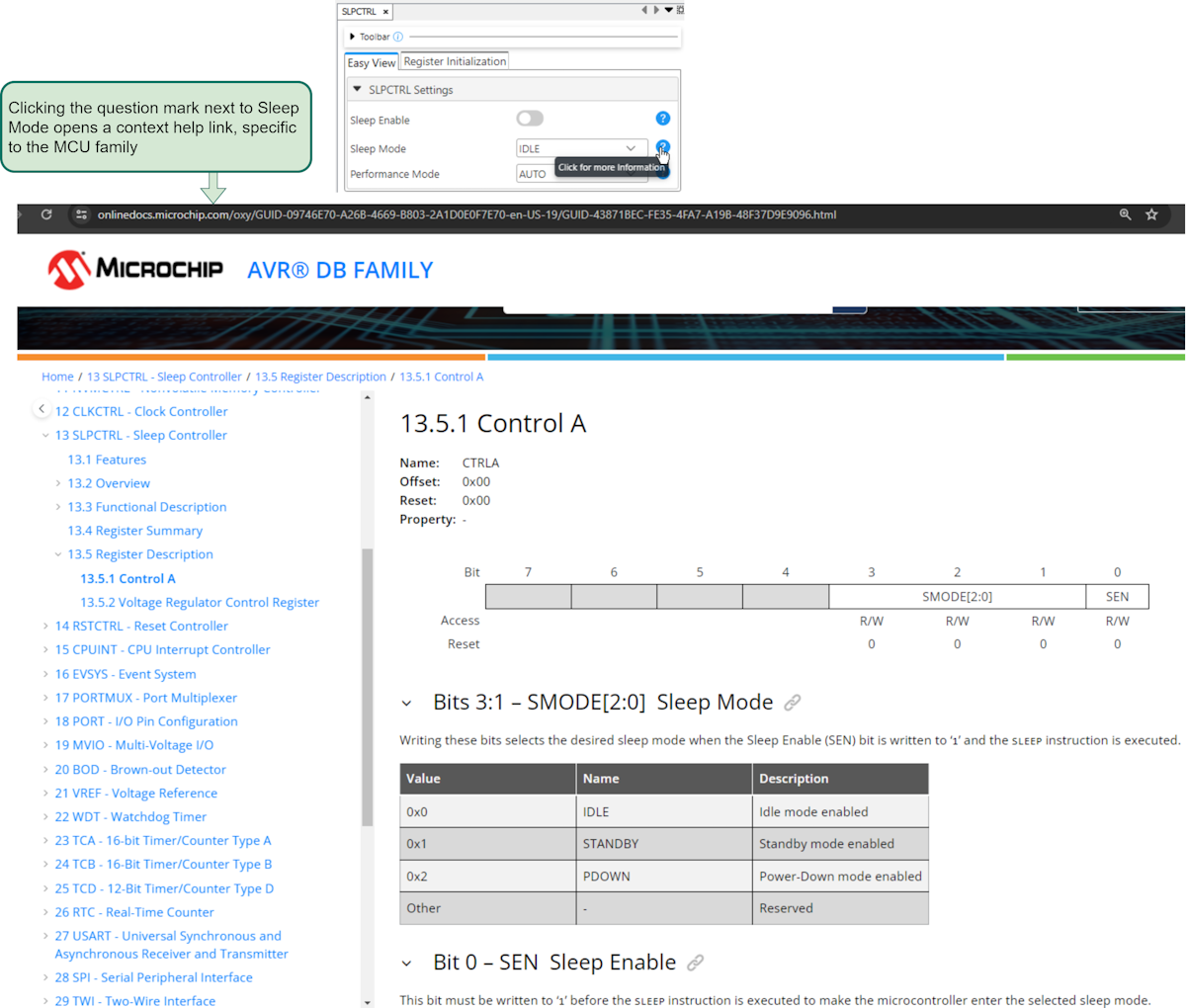
4.2.2.1 Low-Power References and Trainings for AVR
Below is a list of key low-power trainings, mainly for AVR microcontrollers.
Microchip Developer Help has high-level overviews of AVR® Low Power Sleep Modes and the AVR® Device Peripheral Power Reduction Register.
The following documents go into more detail:
- AN2515 AVR® Low-Power Techniques - Discusses several techniques available to help limit the power consumption of AVR microcontrollers. It covers techniques and concepts such as sleep modes, the choice of oscillator and operating frequency, use of the event system, sleepwalking, using Brown-out Detect (BOD) and what to do with unused pins.
- AVR® DA Low-Power Features and Sleep Modes - Presents an overview of the low-power modes and features of the AVR® DA microcontroller family. The current consumption in the different sleep modes is compared with the help of the Power Debugger (ATPOWERDEBUGGER). Furthermore, this application note explains how to modify the Curiosity Nano board to perform power readings, and how to use the Power Debugger and descriptions of the available sleep modes and other power-saving features.
- ADC and Power Optimization with tinyAVR® 0-
and 1- series, and megaAVR® 0-series - Contains five hands-on
applications doing ADC data conversion, with current consumption measured for each
application. The training starts with a simple ADC conversion application. The
following applications introduce different techniques for reducing the current
consumption.Note: The original application note that inspired the training above: AVR4013: picoPower® Basics, video available here.
AVR Sleep
Reference: <avr/sleep.h>: Power Management and Sleep Modes
Using the SLEEP
instruction can significantly reduce the power
consumption of an application. AVR devices can be put into different sleep modes.
Refer to the data sheet for details related to your device.
set_sleep_mode()
(it usually defaults to Idle mode where the
CPU is put to sleep, but all peripheral clocks are still running) and then call
sleep_mode()
. This macro
automatically sets the Sleep Enable (SE) bit, goes to sleep and then clears the bit.
As the sleep_mode()
macro might
cause race conditions in some situations, the individual steps of manipulating the
SE bit and actually issuing the SLEEP
instruction are provided in
the sleep_enable()
, sleep_disable()
and
sleep_cpu()
macros, which
also allows for test-and-sleep scenarios that take care of not missing the interrupt
that will awake the device from sleep.
#include <avr/interrupt.h> #include <avr/sleep.h> ... set_sleep_mode(<mode>); cli(); if (some_condition) { sleep_enable(); sei(); sleep_cpu(); sleep_disable(); } sei();