3.1.2.9 BLE Multiple Advertising Sets
Getting Started with Peripheral Building Blocks
FreeRTOS BLE App Initialize -> BLE Multiple Advertising Sets
Introduction
This document will help users enable BLE Multiple Advertising Sets on the WBZ451 Curiosity board using the MPLAB Code Configurator (MCC). In this application example, two sets of advertisements are implemented. For more details, refer to Section “4.4.2.10 Advertising Sets” of BLUETOOTH CORE SPECIFICATION Version 5.4 | Vol 6, Part B.
Users can choose to just run the precompiled Application Example hex file on the WBZ451 Curiosity board and experience the demo or can go through the steps involved in developing this application from scratch.
These examples each build on top of one another. We strongly recommend that you follow the examples in order, by learning the basic concepts first before progressing to the more advanced topics.
Recommended Reading
Hardware Requirement
Tool | Qty |
---|---|
WBZ451 Curiosity Board | 1 |
Micro USB cable | 1 |
Android/iOS smartphone | 1 |
SDK Setup
Gettting Started with Software DevelopmentSoftware Requirement
Tera TermSmartphone App
Light Blue iOS/Android app available in stores
Programming the Precompiled hex file or Application Example
Programming the hex file using MPLABX IPE
-
Import and program the Precompiled Hex file:
<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\peripheral\two_set_adv\hex\two_set_adv.X.production.signed.hex
-
For more details on the steps, go to Programming A Device .Note: Ensure to choose the correct Device and Tool information
Programming the Application using MPLABX IDE
-
Follow steps mentioned in Running a Precompiled Example
-
Open and program the Application:
<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\peripheral\ two_set_adv\firmware\two_set_adv.X
For more details on how to find the Harmony Content Path, refer to Installing the MCC Pluggin
Demo Description
This application transmits two sets of advertisements: one connectable and the other non-connectable. On reset, this demo will print “2 Set Advertising...” in a terminal emulator like Tera Term. This denotes the start of advertisements.
Testing
- Using a micro USB cable, connect the Debug USB on the Curiosity board to a PC
- Program the precompiled hex file or application example as mentioned
- Open Tera Term and set the “Serial Port” to USB Serial Device and “Speed” to
115200.
For more details on how to set the “Serial Port” and “Speed”, refer to COM Port Setup
- Press the Reset Switch on the Curiosity board. "2 Set Advertising..." should
be displayed in Tera Term.
- Launch the Light Blue mobile app to scan for Advertisements. Device names
"WBZ351_1" and "WBZ351_2" should appear.
Developing the Application from Scratch using MPLAB Code Configurator
-
Create a new MCC Harmony Project
-
To setup the basic components and configuration required to develop this application, import component configuration:
<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\peripheral\two_set_adv\firmware\two_set_adv.X\two_set_adv.mc3
For more details, refer to Import existing App Example ConfigurationNote: Import and Export functionality of the Harmony component configuration will help users to start from a known working setup of the MCC configuration. -
To accept dependencies or satisfiers, select "Yes"
-
Verify if the Project Graph window has all the expected configuration.
Verifying Advertisement
-
Select the BLE Stack component in the Project Graph and configure the following in the Configuration Options panel
- Advertising
Set1
- Advertising Set2
- Advertising
Set1
Generating a Code
For more details on code generation, refer to MPLAB Code Configurator(MCC) Code Generation.
Files and Routines Automatically generated by the MCC
After generating the program source from the MCC interface by clicking Generate Code, the BLE configuration source and header files can then be found in the following project directories.
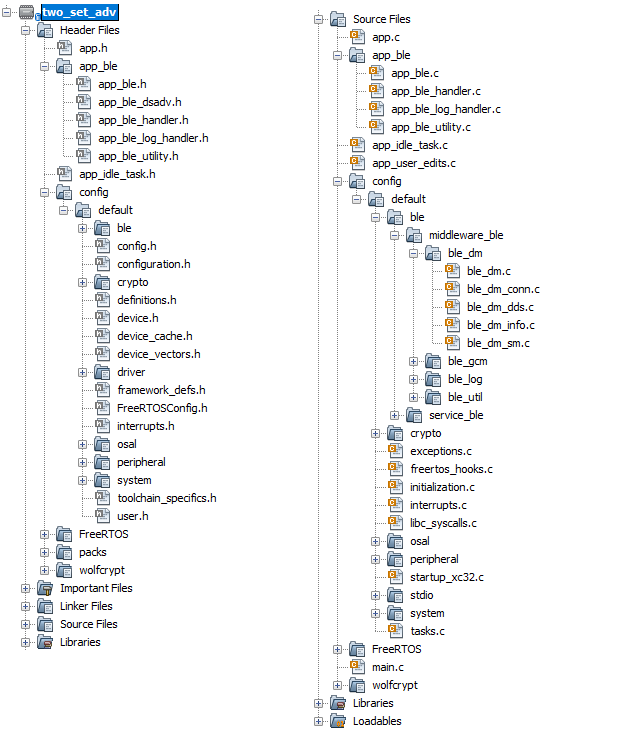
The OSAL, RF System and BLE System initialization routine executed during program initialization can be found in the project file. This initialization routine is automatically generated by the MCC.
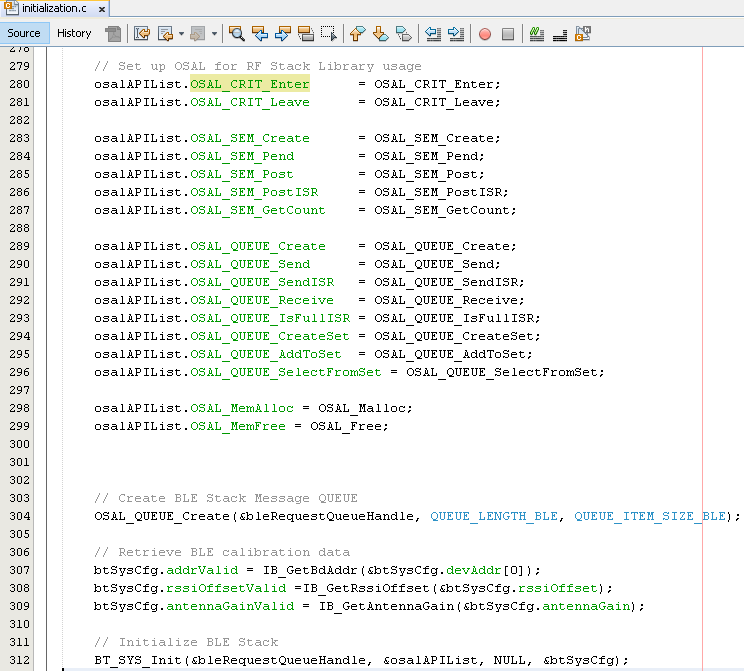
The BLE stack initialization routine executed during Application Initialization can be found in project files. This initialization routine is automatically generated by the MCC. This call initializes and configures the GAP, GATT, SMP, L2CAP and BLE middleware layers.
Autogenerated, advertisement Data Format
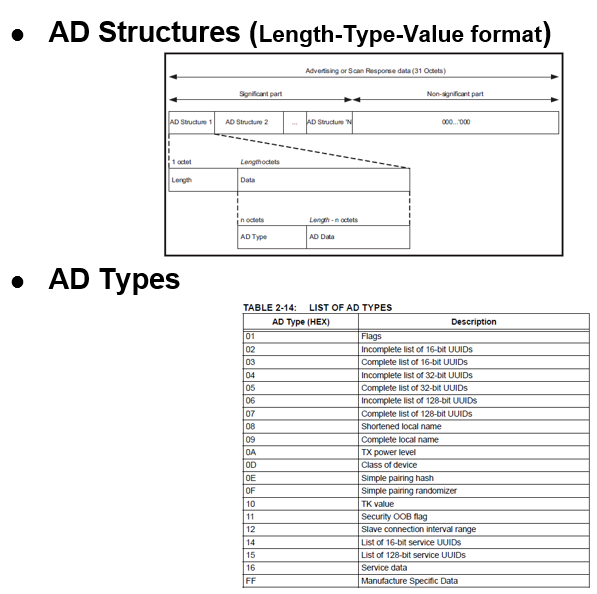
Source Files | Usage |
---|---|
app.c | Application State machine, includes calls for Initialization of all BLE stack (GAP,GATT, SMP, L2CAP) related component configurations |
app_ble.c | Source Code for the BLE stack related component configurations, code related to function calls from app.c |
app_ble_handler.c | All GAP, GATT, SMP and L2CAP Event handlers |
app.c
is autogenerated and has a state
machine-based application code sample. Users can use this template to develop their
own application.Header Files
-
ble_gap.h
contains BLE GAP functions and is automatically included inapp.c
Function Calls
- MCC generates and adds the code to initialize the BLE Stack GAP, GATT, SMP and L2CAP in APP_BleStackInit()
- APP_BleStackInit() is the API that will be called inside the Applications
Initial State APP_STATE_INIT in
app.c
User Application Development
Include
- User Action is required
definitions.h
must be included in all the files where UART will be used to print debug information
definitions.h
is not
specific to just UART but instead must be included in all the application source
files where any peripheral functionality will be exercisedConfigure 2 set of Advertisements
-
APP_BleConfigAdvance();
The following code snippet is to configure Advertising Set 1:
//Configure advertising Set 1
advParams.advHandle = 1; /* Advertising Handle */
advParams.evtProperies = BLE_GAP_EXT_ADV_EVT_PROP_CONNECTABLE_ADV|BLE_GAP_EXT_ADV_EVT_PROP_SCANNABLE_ADV|BLE_GAP_EXT_ADV_EVT_PROP_LEGACY_ADV; /* Advertising Event Properties */
advParams.priIntervalMin = 32; /* Primary Advertising Interval Min */
advParams.priIntervalMax = 32; /* Primary Advertising Interval Max */
advParams.priChannelMap = BLE_GAP_ADV_CHANNEL_ALL; /* Primary Advertising Channel Map */
memset(&advParams.peerAddr, 0x00, sizeof(advParams.peerAddr));
advParams.filterPolicy = BLE_GAP_ADV_FILTER_DEFAULT; /* Advertising Filter Policy */
advParams.txPower = 9; /* Advertising TX Power */
advParams.priPhy = BLE_GAP_PHY_TYPE_LE_1M; /* Primary Advertising PHY */
advParams.secMaxSkip = 0; /* Secondary Advertising Max Skip */
advParams.secPhy = BLE_GAP_PHY_TYPE_LE_1M; /* Secondary Advertising PHY */
advParams.sid = 1; /* Advertising SID */
advParams.scanReqNotifiEnable = false; /* Scan Request Notification Enable */
BLE_GAP_SetExtAdvParams(&advParams, &selectedTxPower);
appAdvData.advHandle = 1;
appAdvData.operation = BLE_GAP_EXT_ADV_DATA_OP_COMPLETE;
appAdvData.fragPreference = BLE_GAP_EXT_ADV_DATA_FRAG_PREF_ALL;
appAdvData.advLen = sizeof(advData);
appAdvData.p_advData=OSAL_Malloc(appAdvData.advLen);
if(appAdvData.p_advData)
{
memcpy(appAdvData.p_advData, advData, appAdvData.advLen); /* Advertising Data */
}
BLE_GAP_SetExtAdvData(&appAdvData);
OSAL_Free(appAdvData.p_advData);
The following code snippet is to configure Advertising Set 2:
//Configure advertising Set 2
advParams.advHandle = 2; /* Advertising Handle */
advParams.evtProperies = BLE_GAP_EXT_ADV_EVT_PROP_LEGACY_ADV; /* Advertising Event Properties */
advParams.priIntervalMin = 32; /* Primary Advertising Interval Min */
advParams.priIntervalMax = 32; /* Primary Advertising Interval Max */
advParams.priChannelMap = BLE_GAP_ADV_CHANNEL_ALL; /* Primary Advertising Channel Map */
memset(&advParams.peerAddr, 0x00, sizeof(advParams.peerAddr));
advParams.filterPolicy = BLE_GAP_ADV_FILTER_DEFAULT; /* Advertising Filter Policy */
advParams.txPower = 9; /* Advertising TX Power */
advParams.priPhy = BLE_GAP_PHY_TYPE_LE_1M; /* Primary Advertising PHY */
advParams.secMaxSkip = 0; /* Secondary Advertising Max Skip */
advParams.secPhy = BLE_GAP_PHY_TYPE_LE_1M; /* Secondary Advertising PHY */
advParams.sid = 2; /* Advertising SID */
advParams.scanReqNotifiEnable = false; /* Scan Request Notification Enable */
BLE_GAP_SetExtAdvParams(&advParams, &selectedTxPower2);
appAdvData.advHandle = 2;
appAdvData.operation = BLE_GAP_EXT_ADV_DATA_OP_COMPLETE;
appAdvData.fragPreference = BLE_GAP_EXT_ADV_DATA_FRAG_PREF_ALL;
appAdvData.advLen = sizeof(advData2);
appAdvData.p_advData=OSAL_Malloc(appAdvData.advLen);
if(appAdvData.p_advData)
{
memcpy(appAdvData.p_advData, advData2, appAdvData.advLen); /* Advertising Data */
}
BLE_GAP_SetExtAdvData(&appAdvData);
OSAL_Free(appAdvData.p_advData);
Start Advertisement in app.c
- BLE_GAP_SetExtAdvEnable(true, 2, extAdvEnableParam);
This API is called in the Applications initialization - APP_STATE_INIT in app.c
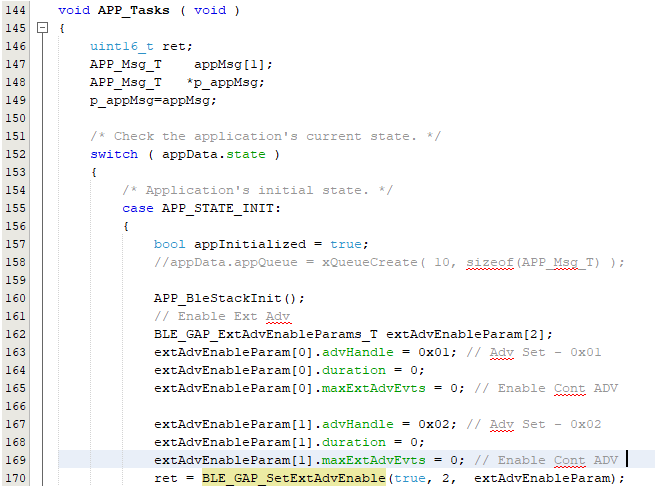