9.5.2.1 dsPIC33C/E/F and dsPIC30 Example
For example:
struct foo {
long long i:40;
int j:16;
char k:8;
} x;
struct bar {
long long I:40;
char J:8;
int K:16;
} y;
struct foo
will have a size of 10 bytes using the
compiler. i
will be allocated at bit offset 0 (through 39). There will
be 8 bits of padding before j
, allocated at bit offset 48. If
j
were allocated at the next available bit offset (40), it would
cross a storage boundary for a 16 bit integer. k
will be allocated
after j
, at bit offset 64. The structure will contain 8 bits of padding
at the end to maintain the required alignment in the case of an array. The alignment is
2 bytes because the largest alignment in the structure is 2 bytes.
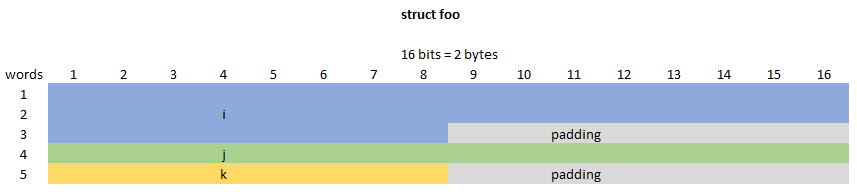
struct bar
will have a size of 8 bytes using the
compiler. I
will be allocated at bit offset 0 (through 39). There is no
need to pad before J because it will not cross a storage boundary for a
char
. J
is allocated at bit offset 40.
K
can be allocated starting at bit offset 48, completing the
structure without wasting any space.

Unnamed bitfields may be declared to pad out unused space between active bits in control registers. For example:
struct foo {
unsigned int lo : 1;
unsigned int : 6;
unsigned int hi : 1;
} x;