Add Code to Toggle LED with a Delay
Previously code was added to the main source file to enable a pull-up on PC7 for better switch response and the user LED was turned on. Now code is added to toggle the LED on and off. Due to the speed of the device this could be imperceptible unless a delay is added to slow down the toggle. Information on the delay used can be found at:
www.nongnu.org/avr-libc/user-manual/group__util__delay.html
A #define
for the CPU frequency needs to be added as well as a
#include
for delay support.
To determine the F_CPU
for the Curiosity Nano, open the View IO window
again and perform a debug run of the code to get live values in the window. Once the
code is in the while(1)
loop, Pause and look at CLKCTRL to find the
value.
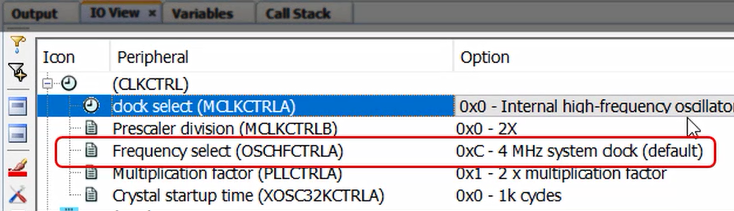
The updated code will be as shown below.
#include <avr/io.h>
#define F_CPU 4000000UL
#include <util/delay.h>
int main(void) {
PORTC.PIN7CTRL = PORT_PULLUPEN_bm; /* Enable PC7 Pullup */
PORTC.DIR = PIN6_bm; /* Turn on LED */
while (1) {
PORTC.OUTTGL = PIN6_bm; /* Toggle LED on/off */
_delay_ms(500); /* wait between toggles */
}
}
Plotting the GPIO pins again, you can see PC7 (GPIO 0) in blue with different sized pulses from button presses and PC6 (GPIO 1) in green with pulses from toggling.
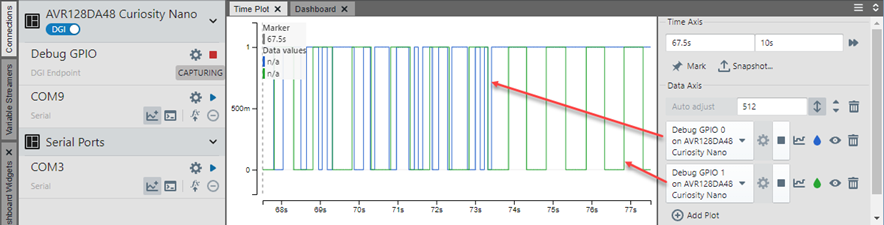