6.4.5 NVMREG Write to Program Memory
- Load the address of the row to be programmed into NVMADRH:NVMADRL.
- Load each write latch with data.
- Initiate a programming operation.
- Repeat steps 1 through 3 until all data is written.
Before writing to program memory, the word(s) to be written must be erased or previously unwritten. Program memory can only be erased one row at a time. No automatic erase occurs upon the initiation of the write.
Program memory can be written one or more words at a time. The maximum number of words written at one time is equal to the number of write latches. See Figure 6-4 for more details.
The write latches are aligned to the Flash row address boundary defined by the upper ten bits of NVMADRH:NVMADRL (NVMADRH[6:0]:NVMADRL[7:5]), with the lower five bits of NVMADRL (NVMADRL[4:0]) determining the write latch being loaded. Write operations do not cross these boundaries. At the completion of a program memory write operation, the data in the write latches is reset to contain 0x7FFF.
1
. When the last word to be loaded into the write latch is ready,
the LWLO bit is cleared and the unlock sequence executed. This initiates the programming
operation, writing all the latches into Flash program memory.- Set the WREN bit of the NVMCON1 register.
- Clear the NVMREGS bit of the NVMCON1 register.
- Set the LWLO bit of the NVMCON1
register. When the LWLO bit of the NVMCON1 register is ‘
1
’, the write sequence will only load the write latches and will not initiate the write to Flash program memory. - Load the NVMADRH:NVMADRL register pair with the address of the location to be written.
- Load the NVMDATH:NVMDATL register pair with the program memory data to be written.
- Execute the unlock sequence. The write latch is now loaded.
- Increment the NVMADRH:NVMADRL register pair to point to the next location.
- Repeat steps 5 through 7 until all but the last write latch has been loaded.
- Clear the LWLO bit of the NVMCON1
register. When the LWLO bit of the NVMCON1 register is ‘
0
’, the write sequence will initiate the write to Flash program memory. - Load the NVMDATH:NVMDATL register pair with the program memory data to be written.
- Execute the unlock sequence. The entire program memory latch content is now written to Flash program memory.
An example of the complete write sequence is shown in Writing to Program Flash Memory. The initial address is loaded into the NVMADRH:NVMADRL register pair; the data is loaded using indirect addressing.
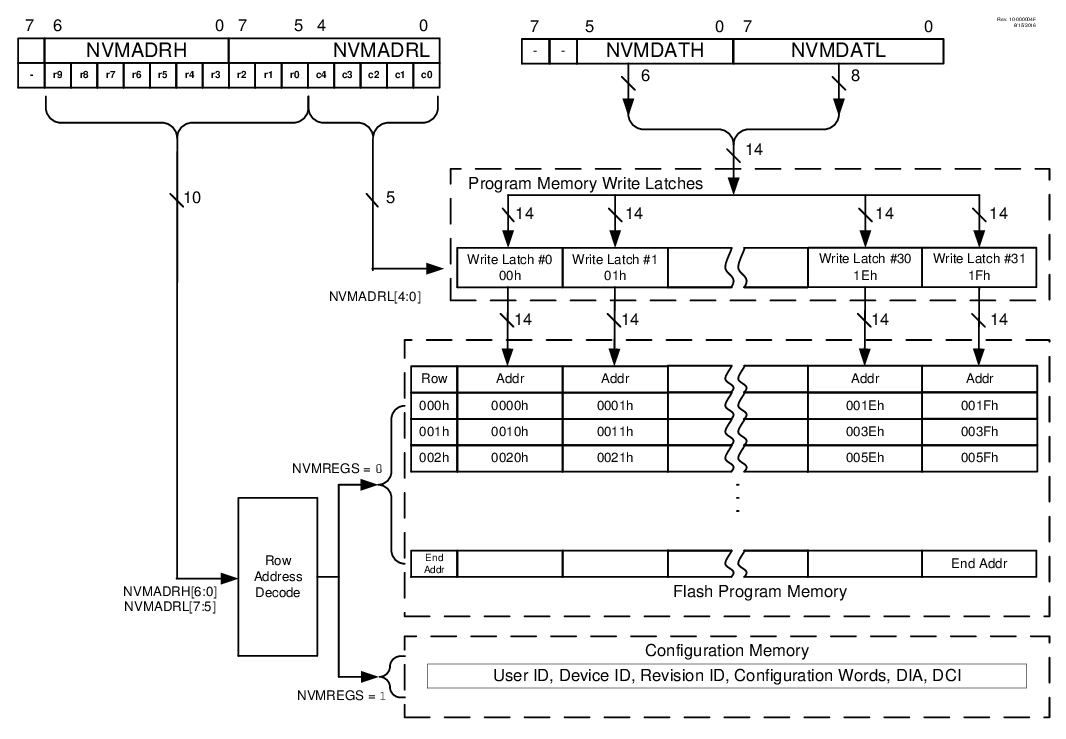
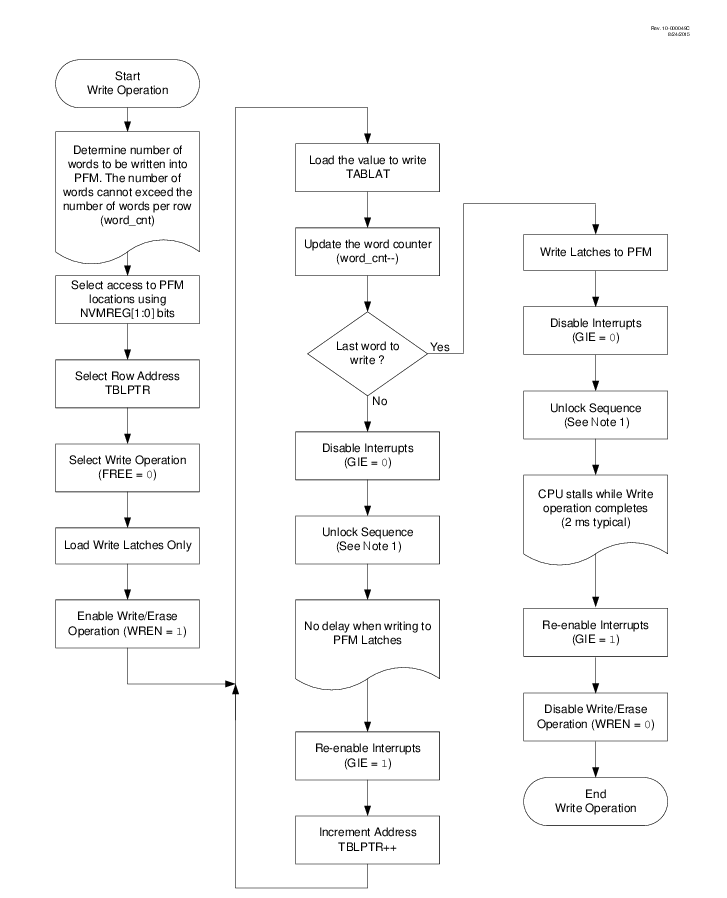
- See Figure 6-2 for an example of the unlock sequence.
Writing to Program Flash Memory
; This write routine assumes the following: ; 1. 64 bytes of data are loaded, starting at ; the address in DATA_ADDR ; 2. Each word of data to be written is made up of two adjacent : bytes in DATA_ADDR,stored in little endian format ; 3. A valid starting address (the Least Significant bits = 00000) : is loaded in ADDRH:ADDRL ; 4. ADDRH and ADDRL are located in common RAM (locations 0x70-0x7F) ; 5. NVM interrupts are not taken into account BANKSEL NVMADRH MOVF ADDRH,W MOVWF NVMADRH ; Load initial address MOVF ADDRL,W MOVWF NVMADRL MOVLW LOW DATA_ADDR ; Load initial data address MOVWF FSR0L MOVLW HIGH DATA_ADDR MOVWF FSR0H BCF NVMCON1,NVMREGS ; Set Program Flash as write location BSF NVMCON1,WREN ; Enable writes BSF NVMCON1,LWLO ; Load only write latches LOOP MOVIW FSR0++ MOVWF NVMDATL ; Load first data byte MOVIW FSR0++ MOVWF NVMDATH ; Load second data byte MOVF NVMADRL,W XORLW 0x1F ; Check if lower bits of address ; are 00000 ANDLW 0x1F ; and if on last of 32 addresses BTFSC STATUS,Z ; Last of 32 words? GOTO START_WRITE ; If so, go write latches into memory CALL UNLOCK_SEQ ; If not, go load latch INCF NVMADRL,F ; Increment address GOTO LOOP START_WRITE BCF NVMCON1,LWLO ; Latch writes complete, ; Now write memory CALL UNLOCK_SEQ ; Perform required unlock sequence BCF NVMCON1,WREN ; Disable writes UNLOCK_SEQ MOVLW 55h BCF INTCON,GIE ; Disable interrupts MOVWF NVMCON2 ; Begin unlock sequence MOVLW AAh MOVWF NVMCON2 BSF NVMCON1,WR BSF INTCON,GIE ; Re-enable interrupts ; Unlock sequence complete return