Line Buffer
(Ask a Question)The LineBuffer
class implements the line buffer structure that is commonly
seen in image convolution (filtering) operations, where a filter kernel is "slided" over an
input image and is applied on a local window (e.g., a square) of pixels at every sliding
location. As the filter is slided across the image, the line buffer is fed with a new pixel
at every new sliding location while retaining the pixels of the previous image rows that
can be covered for the sliding window. The public interface of the
LineBuffer
class is shown below,
template <typename PixelType, unsigned ImageWidth, unsigned WindowSize>
class LineBuffer {
public:
PixelType window[WindowSize][WindowSize];
void ShiftInPixel(PixelType input_pixel);
};
Below shows an example usage of the LineBuffer
class:
- Instantiate the line buffer in your C++
code, with template arguments being the pixel data type, input image width, and sliding
window size. The window maintained by the line buffer assumes a square
WindowSize x WindowSize
window. If you are instantiating the line buffer inside a pipelined function (accepting a new pixel in every function call), you will need to add 'static' to make the line buffer static.static hls::LineBuffer<unsigned char, ImageWidth, WindowSize> line_buffer;
- Shift in a new pixel by calling the
ShiftInPixel
method:line_buffer.ShiftInPixel(input_pixel);
- Then your filter can access any pixels
in the
window
by:line_buffer.window[i][j]
window
is being updated
after each call of ShiftInPixel
. You will notice that the window
can contain out-of-bound pixels at certain sliding locations.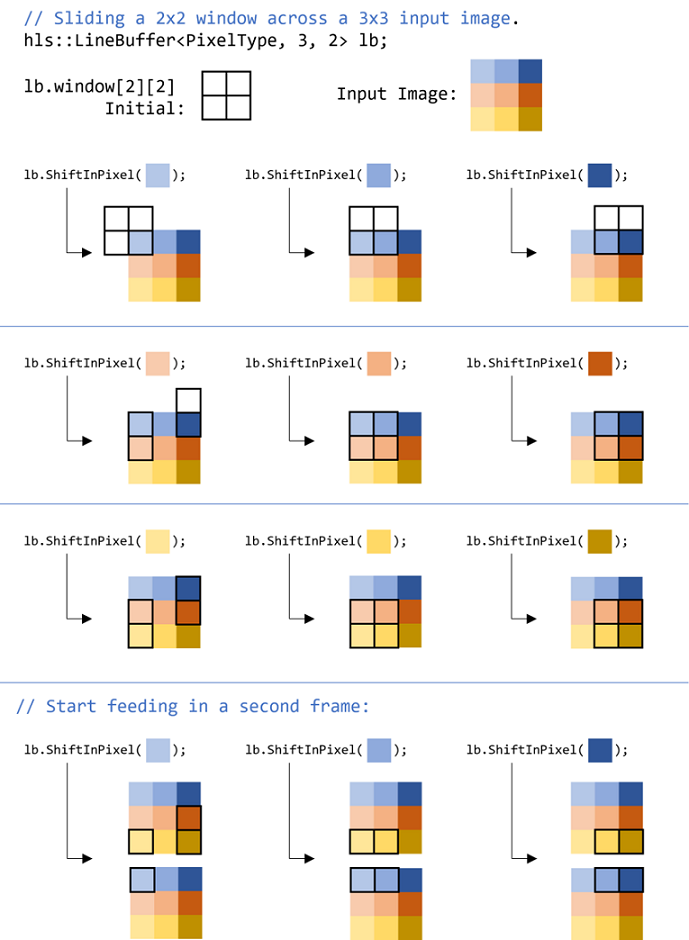
For more details about when/why to use the LineBuffer
class, see Inferring a Line Buffer.
Error Correction Code for Line Buffer
SmartHLS™ also offers an Error Correction Code (ECC) version for Line Buffer. ECC
feature is enabled for the private class member prev_rows[]
(see Inferring a Line Buffer in Optimization Guide for more
details). The public interface of LineBuffer_ECC
class is shown
below:
template <typename PixelType, unsigned ImageWidth, unsigned WindowSize, bool SB_WRITE_BACK = false, bool DB_OVERRIDE = false, PixelType DB_DEFAULT = PixelType()> class LineBuffer_ECC{ public: PixelType window[WindowSize][WindowSize]; void ShiftInPixel(PixelType input_pixel); int sb_count(); int db_count(); int reset_counters(); void scrub(); };
LineBuffer_ECC
uses the following additional template parameters to
configure the memory and error handling:SB_WRITE_BACK
- If true, when a single-bit error is detected, the corrected value is
immediately written-back to correct the corrupted data in the memory.Warning: Enabling immediate write-back using
SB_WRITE_BACK
can affect the performance since the load operation can invoke an immediate store to the same address. This should be taken into consideration if the latency is critical (e.g. in a loop pipeline). DB_OVERRIDE
- If true, instead of using the corrupted data when double-bit error is detected, a default value is used.
DB_DEFAULT
- A default value is used when double-bit error is detected.
The following table outlines the additional API functions in
LineBuffer_ECC
.
Class Method | Description |
---|---|
int sb_count() | Return the number of single-bit error detected and corrected. |
int db_count() | Return the number of double-bit error detected. |
void reset_counters() | Reset both single-bit and double-bit counters to 0. |
void scrub() | Read the memory element-by-element and write them back. Note that
scrub() automatically calls
reset_counters() to reset the error counters. |